1. 二叉树之层序遍历
1.1 144-二叉树的前序遍历
144
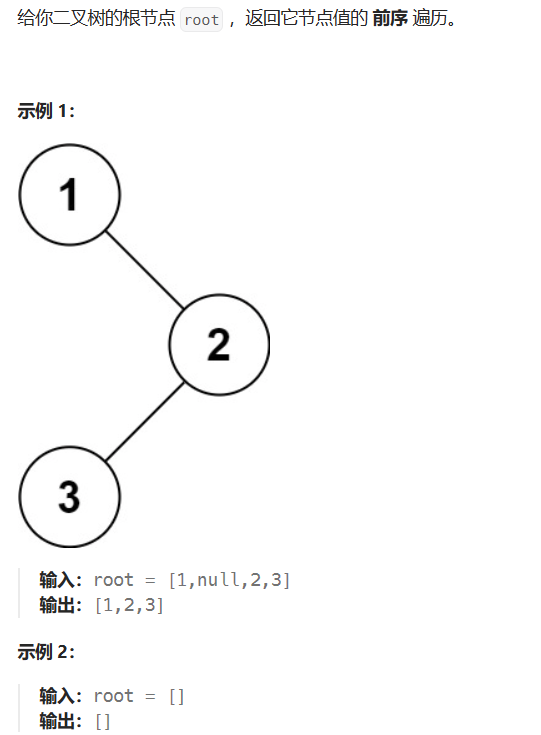
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
|
class Solution { public: void Recursion(TreeNode* root,vector<int>& ans) { if(root == nullptr) return; ans.push_back(root->val); Recursion(root->left,ans); Recursion(root->right,ans); } vector<int> preorderTraversal(TreeNode* root) { vector<int> ans; Recursion(root,ans); return ans; } };
|
迭代难度更大
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Solution { public: vector<int> preorderTraversal(TreeNode* root) { vector<int> ans; stack<TreeNode*> st; TreeNode* cur = root; while(cur || !st.empty()) { while(cur) { ans.push_back(cur->val); st.push(cur); cur = cur->left; } TreeNode* tmp = st.top(); st.pop(); cur = tmp->right; } return ans; } };
|
1.2 94-二叉树的中序遍历
94
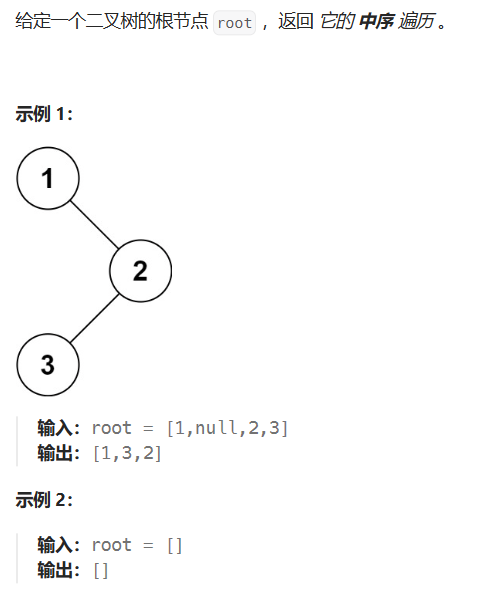
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: void Recursion(TreeNode* root,vector<int>& ans) { if(root == nullptr) return; Recursion(root->left,ans); ans.push_back(root->val); Recursion(root->right,ans); } vector<int> inorderTraversal(TreeNode* root) { vector<int> ans; Recursion(root,ans); return ans; } };
|
迭代法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Solution { public: vector<int> inorderTraversal(TreeNode* root) { stack<TreeNode*> st; vector<int> ans; TreeNode* cur = root; while(cur || !st.empty()) { while(cur) { st.push(cur); cur = cur->left; } TreeNode* tmp = st.top(); ans.push_back(tmp->val); st.pop(); cur = tmp->right; } } };
|
1.3 145-二叉树的后序遍历
145
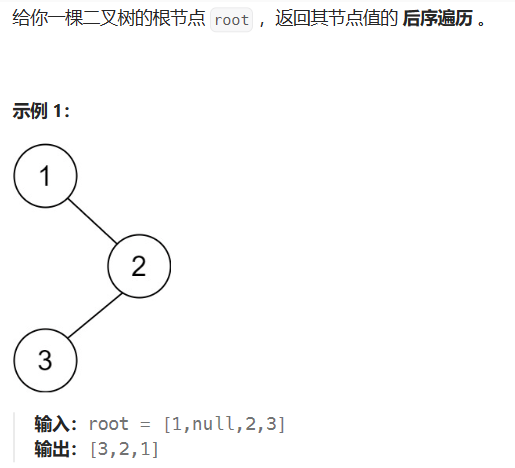
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Solution { public: void Recursion(TreeNode*& root,vector<int>& ans) { if(root==nullptr) return; Recursion(root->left,ans); Recursion(root->right,ans); ans.push_back(root->val); } vector<int> postorderTraversal(TreeNode* root) { vector<int> ans; Recursion(root,ans); return ans; } };
|
迭代法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Solution { public: vector<int> postorderTraversal(TreeNode* root) { vector<int> ans; stack<TreeNode*> st; TreeNode* cur = root; TreeNode* prev = nullptr; while(cur || !st.empty()) { while(cur) { st.push(cur); cur=cur->left; } TreeNode* top = s.top(); if(!top->right || top->right == prev) { ans.push_back(top->val); prev = top; st.pop(); } else cur = top->right; } return ans; } };
|
1.4 102-二叉树的层序遍历
102
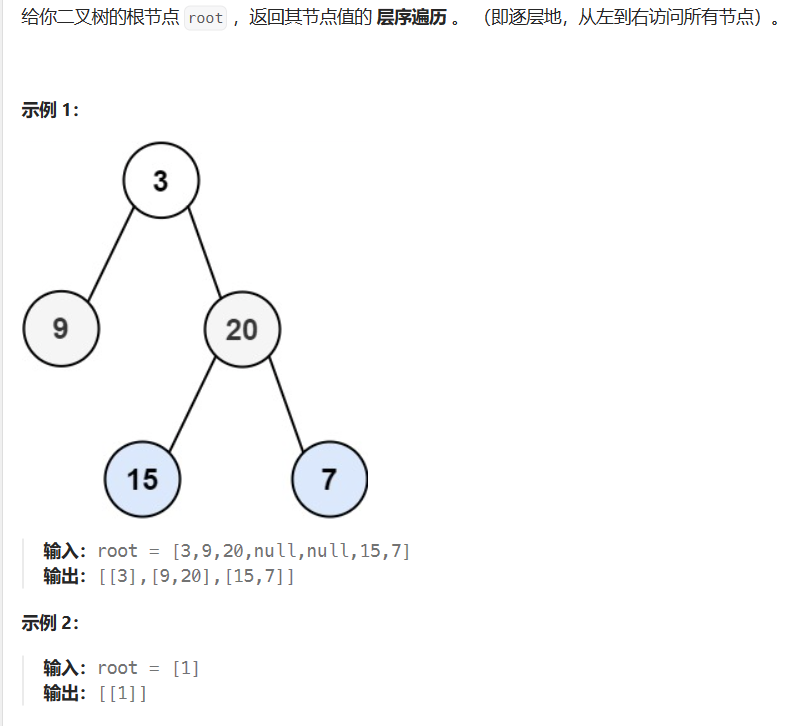
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Solution { public: void Recursion(vector<vector<int>>& ans,TreeNode* root,int level) { if(!root) return; if(ans.size() <= level) ans.push_back(vector<int>()); ans[level].push_back(root->val); Recursion(ans,root->left,level+1); Recursion(ans,root->right,level+1); } vector<vector<int>> levelOrder(TreeNode* root) { vector<vector<int>> ans; Recursion(ans,root,0); return ans; } };
|
1.5 107-二叉树的层序遍历II
107
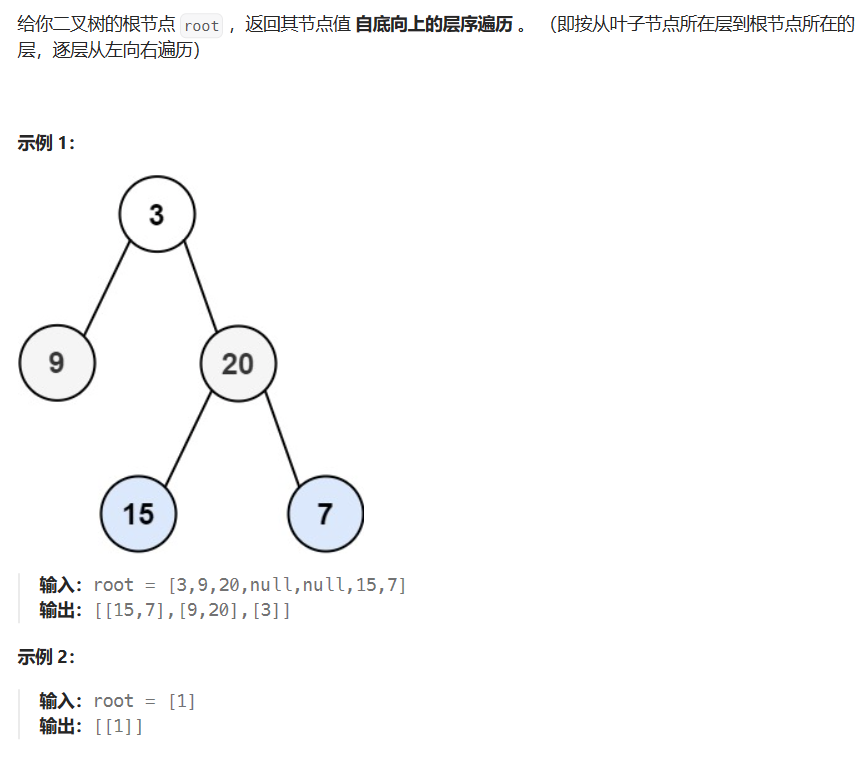
上一题reverse一下就可以了
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class Solution { public: void Recursion(vector<vector<int>>& ans,TreeNode* root,int level) { if(!root) return; if(ans.size() <= level) ans.push_back(vector<int>()); ans[level].push_back(root->val); Recursion(ans,root->left,level+1); Recursion(ans,root->right,level+1); } vector<vector<int>> levelOrderBottom(TreeNode* root) { vector<vector<int>> ans; Recursion(ans,root,0); reverse(ans.begin(),ans.end()); return ans; } };
|
也引入一下这题(上一题)的非递归写法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| class Solution { public: vector<vector<int>> levelOrderBottom(TreeNode* root) { queue<TreeNode*> que; if (root != NULL) que.push(root); vector<vector<int>> result; while (!que.empty()) { int size = que.size(); vector<int> vec; for (int i = 0; i < size; i++) { TreeNode* node = que.front(); que.pop(); vec.push_back(node->val); if (node->left) que.push(node->left); if (node->right) que.push(node->right); } result.push_back(vec); } reverse(result.begin(), result.end()); return result;
} };
|
1.6 199-二叉树的右视图
199
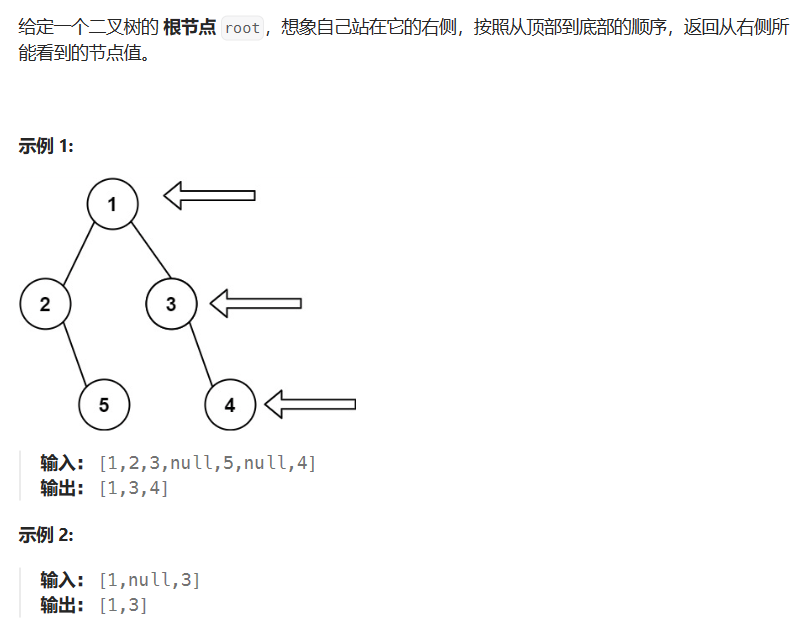
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| class Solution { public: vector<int> rightSideView(TreeNode* root) { queue<TreeNode*> que; vector<int> ans; if(root) que.push(root); while(!que.empty()) { int size = que.size(); for(int i = 0; i < size;i++) { TreeNode* tmp = que.front(); que.pop(); if(i == (size-1)) ans.push_back(tmp->val); if(tmp->left) que.push(tmp->left); if(tmp->right) que.push(tmp->right); } } return ans; } };
|
1.7* 637-二叉树的层平均值
637
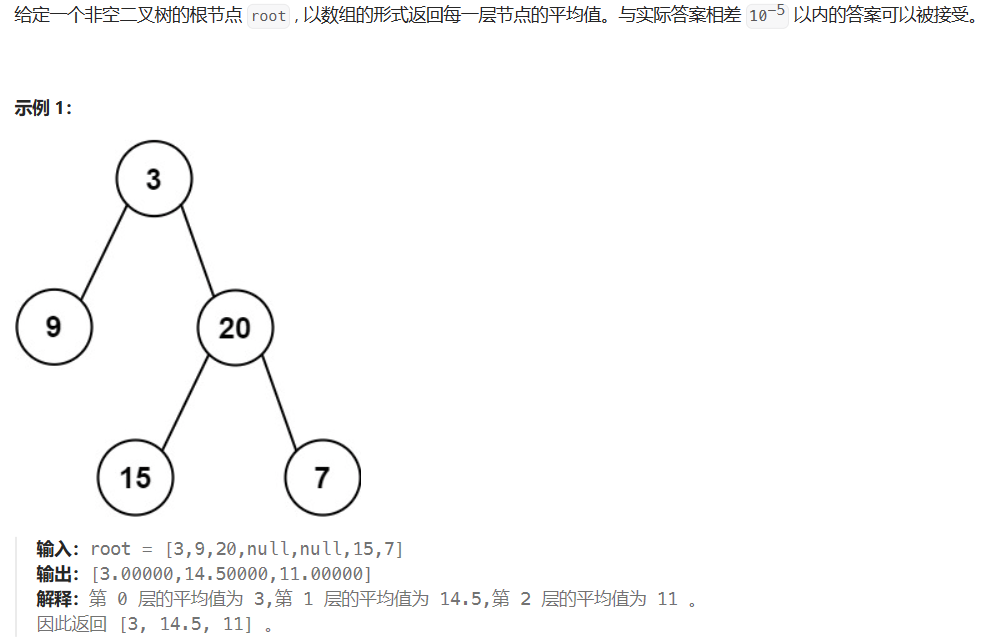
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Solution { public: vector<double> averageOfLevels(TreeNode* root) { queue<TreeNode*> que; vector<double> ans; if(root) que.push(root); while(!que.empty()) { int size = que.size(); double sum = 0; for(int i = 0; i < size; ++i) { TreeNode* tmp = que.front(); que.pop(); sum+=tmp->val;
if(tmp->left) que.push(tmp->left); if(tmp->right) que.push(tmp->right); } ans.push_back(sum/size); } return ans; } };
|
1.8* 429-N叉树的层序遍历
429
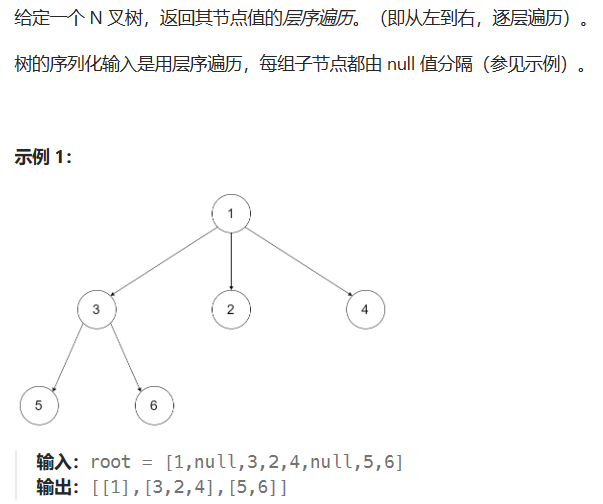
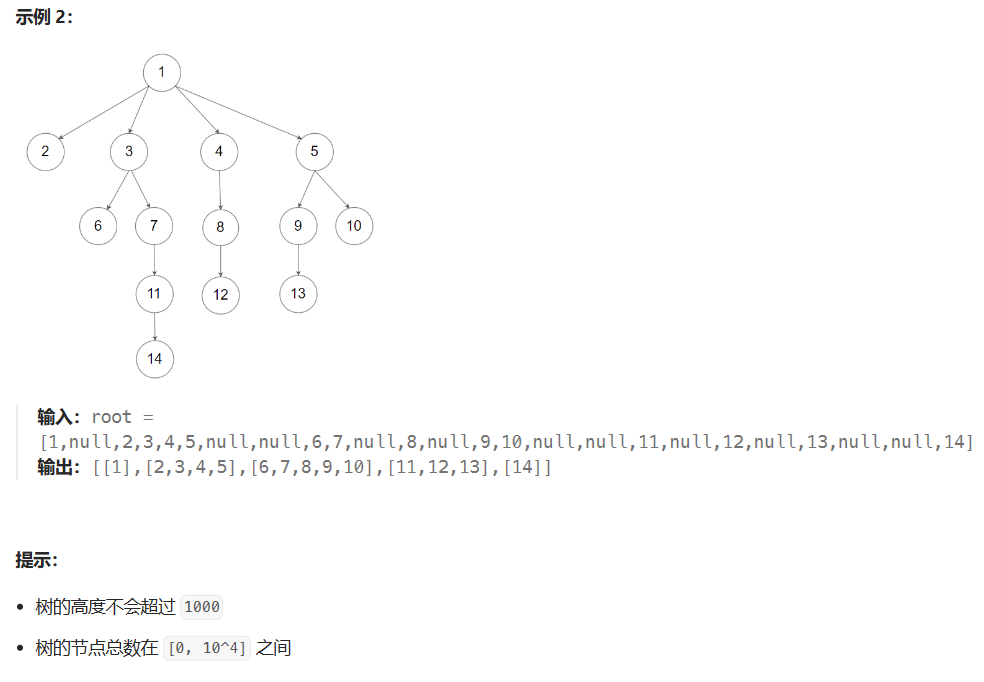
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
|
class Solution { public: vector<vector<int>> levelOrder(Node* root) { queue<Node*> que; vector<vector<int>> ans; if(root) que.push(root); while(!que.empty()) { int size = que.size(); vector<int> tmp_v; for(int i = 0; i < size; ++i) { Node* node = que.front(); que.pop(); tmp_v.push_back(node->val);
for(int j = 0; j < node->children.size(); ++j) if(node->children[j]) que.push(node->children[j]); } ans.push_back(tmp_v); } return ans; } };
|
1.9 515-在每个树行中找最大值
515
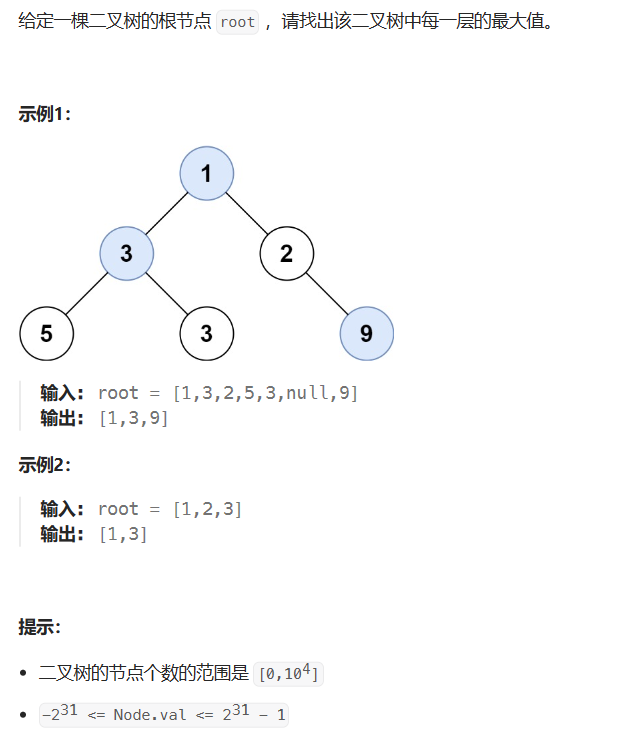
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Solution { public: vector<int> largestValues(TreeNode* root) { vector<int> ans; queue<TreeNode*> que; if(root) que.push(root); while(!que.empty()) { int size = que.size(); int max = INT_MIN; for(int i = 0; i < size;++i) { TreeNode* tmp = que.front(); que.pop(); if(tmp->val>max) max = tmp->val; if(tmp->left) que.push(tmp->left); if(tmp->right) que.push(tmp->right); } ans.push_back(max); } return ans; } };
|
1.10* 116-填充每个节点的下一个右侧节点指针
116
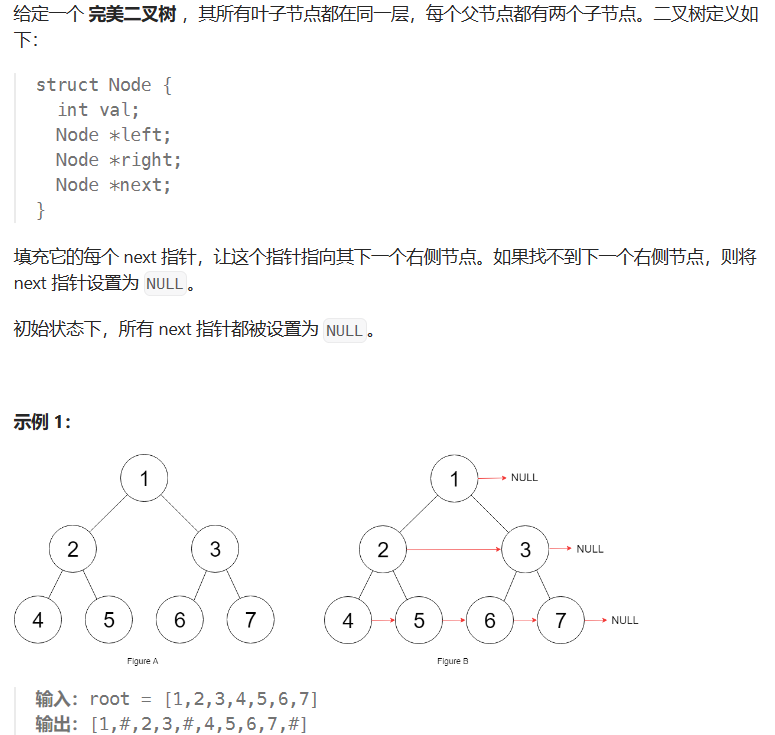

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
|
class Solution { public: Node* connect(Node* root) { if(!root) return nullptr; Node* leftest = root; while(leftest->left) { Node* cur = leftest; while(cur) { cur->left->next = cur->right; if(cur->next) cur->right->next = cur->next->left; cur = cur->next; } leftest = leftest->left; } return root; } };
|
若使用普通的层序遍历那么空间复杂度会达到N
而这种方式空间复杂度为1
1.11 117-填充每个节点的下一个右侧节点指针II
117
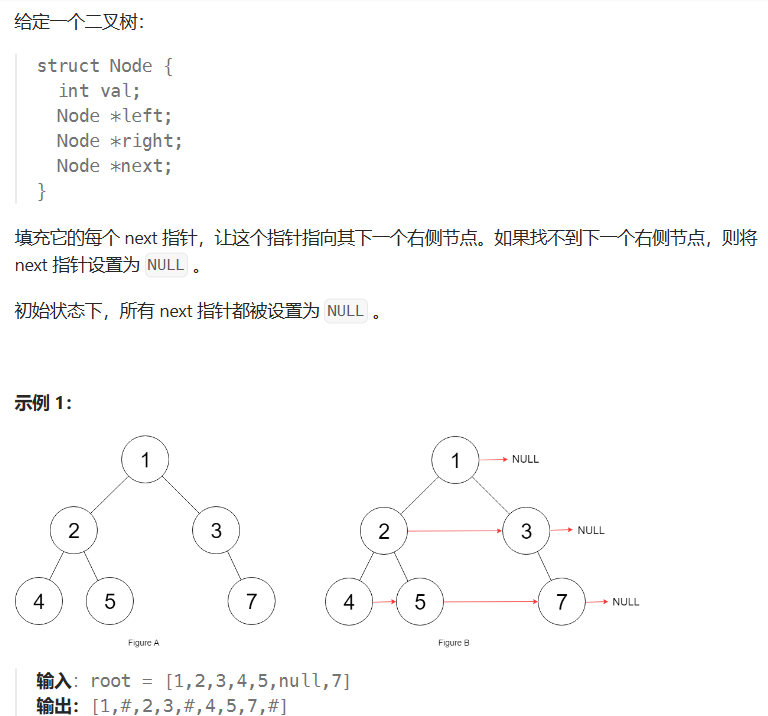

上一题是完全二叉树
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
|
class Solution { public: Node* connect(Node* root) { if(root && (root->left || root->right)) { if(root->left && root->right) root->left->next = root->right;
Node* child = root->right ? root->right : root->left; Node* brodady = root->next; while(brodady && !(brodady->left||brodady->right)) brodady = brodady->next;
child->next = brodady ? (brodady->left ? brodady->left : brodady->right) : nullptr; connect(root->right); connect(root->left); } return root; } };
|
1.12 104-二叉树的最大深度
104
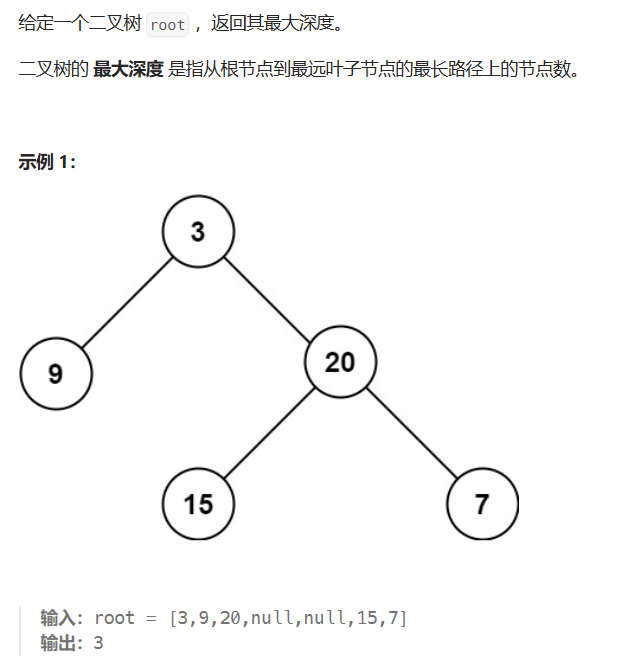
1 2 3 4 5 6 7 8
| class Solution { public: int maxDepth(TreeNode* root) { if(!root) return 0; return max(maxDepth(root->left), maxDepth(root->right)) + 1; } };
|
1.13 111-二叉树的最小深度
111
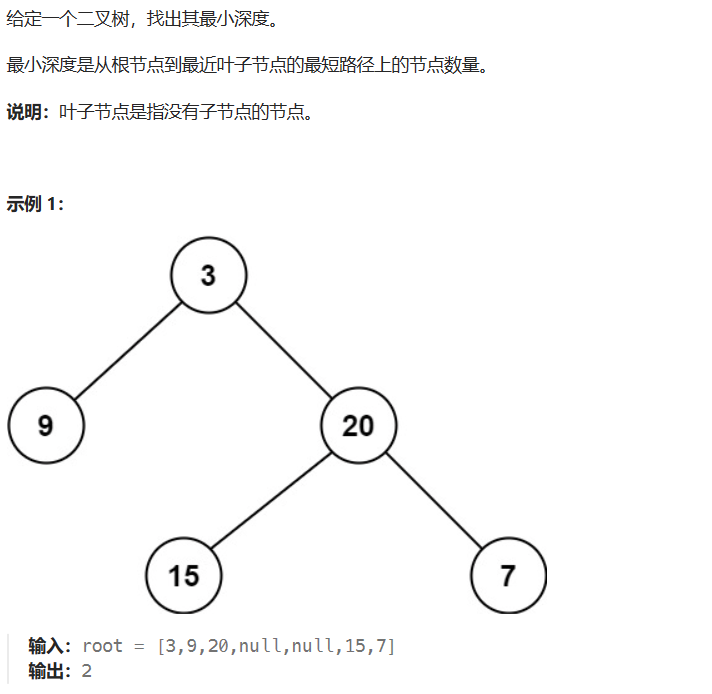
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: int minDepth(TreeNode* root) { if(!root) return 0; if(!root->left && !root->right) return 1; int min_depth = INT_MAX; if(root->left) min_depth = min(minDepth(root->left),min_depth); if(root->right) min_depth = min(minDepth(root->right),min_depth); return min_depth+1; } };
|
2. 二叉树之常见算法
2.1 226-翻转二叉树
226
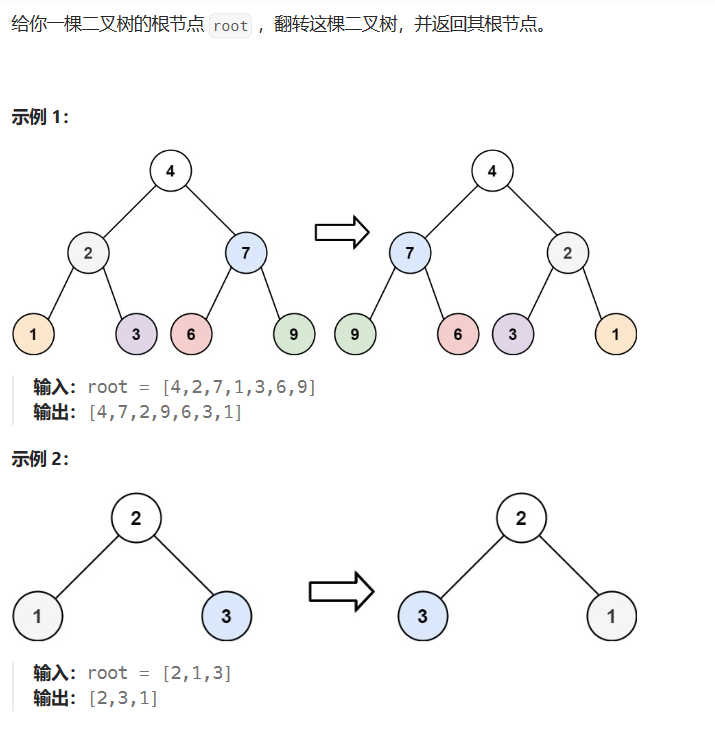
1 2 3 4 5 6 7 8 9 10 11
| class Solution { public: TreeNode* invertTree(TreeNode* root) { if(!root) return nullptr; swap(root->left,root->right); invertTree(root->left); invertTree(root->right); return root; } };
|
2.2 101-对称二叉树
101
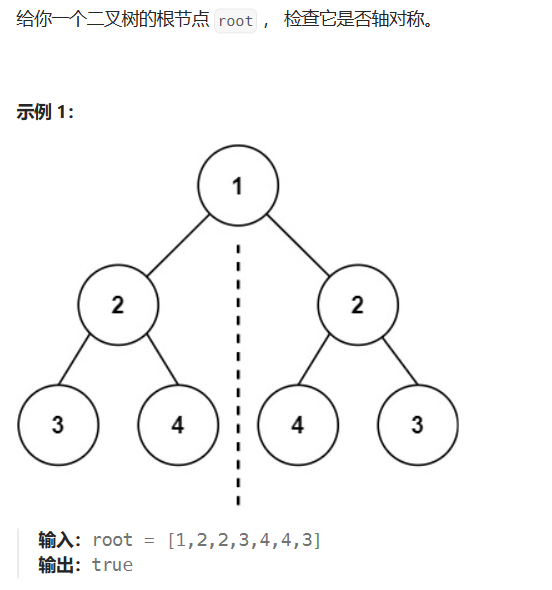
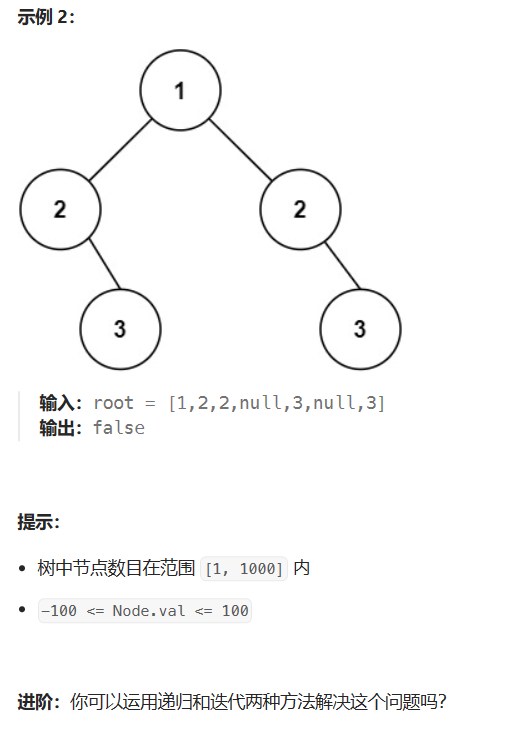
递归
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Solution { public: bool Recursion(TreeNode* left,TreeNode* right) { if(!left&&right || left&&!right) return false; if(!left && !right) return true; if(left->val != right->val) return false; return Recursion(left->left,right->right) && Recursion(left->right,right->left); } bool isSymmetric(TreeNode* root) { return Recursion(root->left,root->right); } };
|
迭代(栈)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Solution { public: bool isSymmetric(TreeNode* root) { stack<TreeNode*> st; st.push(root->left); st.push(root->right); while (!st.empty()) { TreeNode* leftNode = st.top(); st.pop(); TreeNode* rightNode = st.top(); st.pop(); if (!leftNode && !rightNode) continue; if ((!leftNode || !rightNode || (leftNode->val != rightNode->val))) return false; st.push(leftNode->left); st.push(rightNode->right); st.push(leftNode->right); st.push(rightNode->left); } return true; } };
|
2.3* 222-完全二叉树的节点个数
222
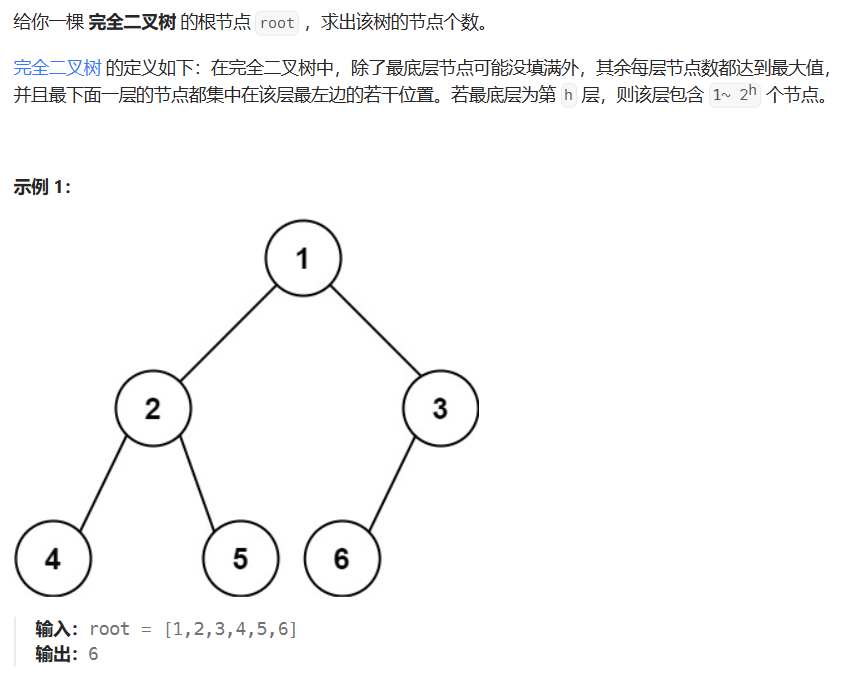
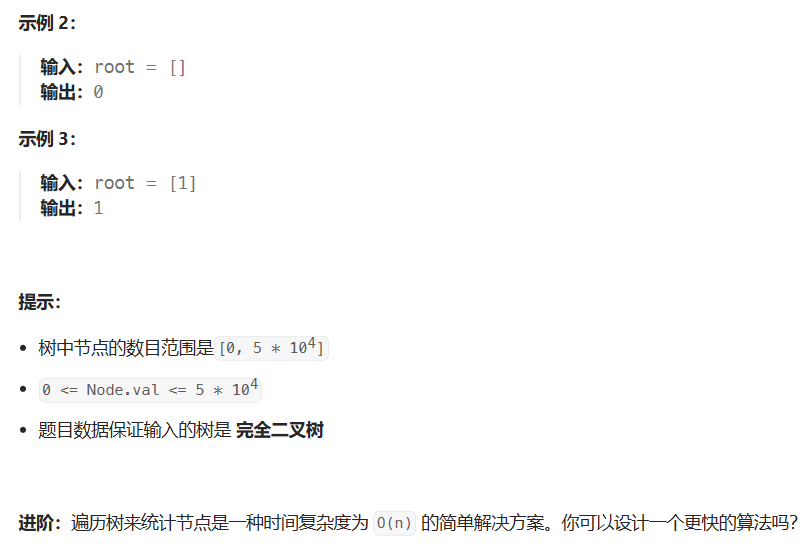
递归遍历 O(N)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: void Recursion(TreeNode* root,int& count) { if(!root) return; count++; Recursion(root->left,count); Recursion(root->right,count); } int countNodes(TreeNode* root) { int count = 0; Recursion(root,count); return count; } };
|
精简版递归
1 2 3 4 5 6 7 8 9
| class Solution { public: int countNodes(TreeNode* root) { if (root == NULL) return 0; return 1 + countNodes(root->left) + countNodes(root->right); } };
|
最优
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| class Solution { public: int countNodes(TreeNode* root) { if (!root) return 0; int level = 0; TreeNode* cur = root; while (cur->left) { level++; cur = cur->left; } int low = 1 << level; int high = (1 << (level + 1)) - 1; while (low < high) { int mid = (high - low + 1) / 2 + low; if (exists(root, level, mid)) low = mid; else high = mid - 1; } return low; }
bool exists(TreeNode* root, int level, int mid) { int bits = 1 << (level - 1); TreeNode* cur = root; while (cur && bits > 0) { if (bits & mid) cur = cur->right; else cur = cur->left; bits >>= 1; } return cur != nullptr; } };
|
时间复杂度:O(logN*logN)
空间复杂度:O(1)
2.4 110-平衡二叉树
110
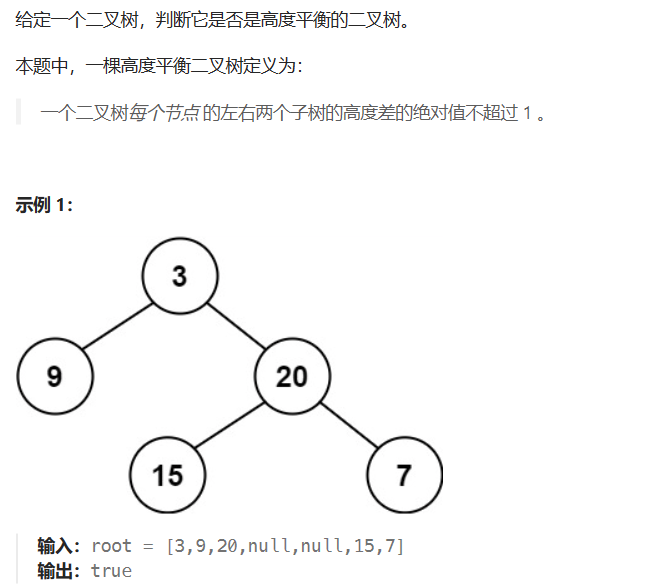
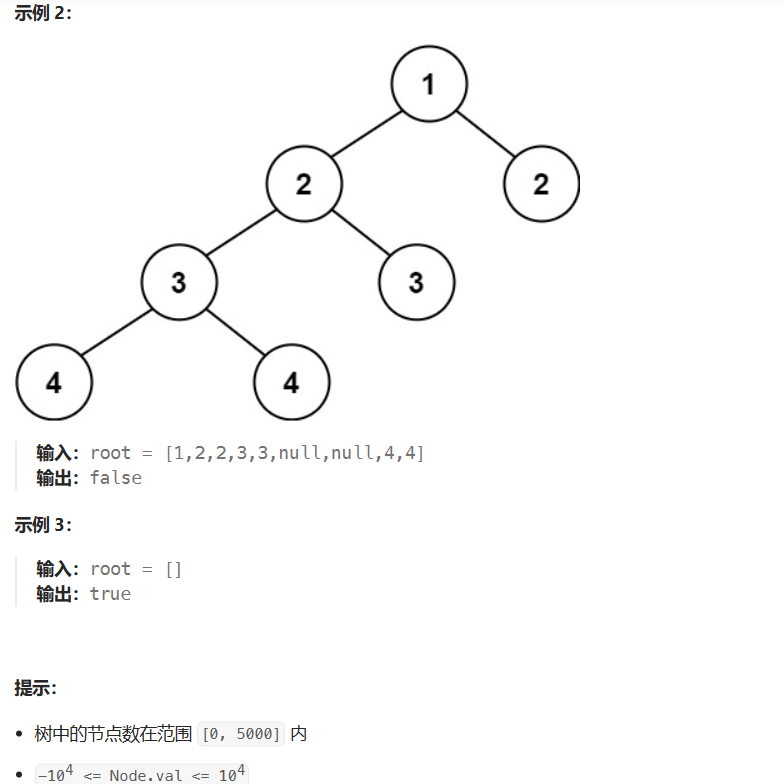
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class Solution { public: int Recursion(TreeNode* root) { if(!root) return 0; int left = Recursion(root->left); if(left == -1) return -1; int right = Recursion(root->right); if(right == -1) return -1; if(abs(left-right) > 1) return -1; return left>right?left+1:right+1; } bool isBalanced(TreeNode* root) { if(Recursion(root) == -1) return false; return true; } };
|
2.5 257-二叉树的所有路径
257
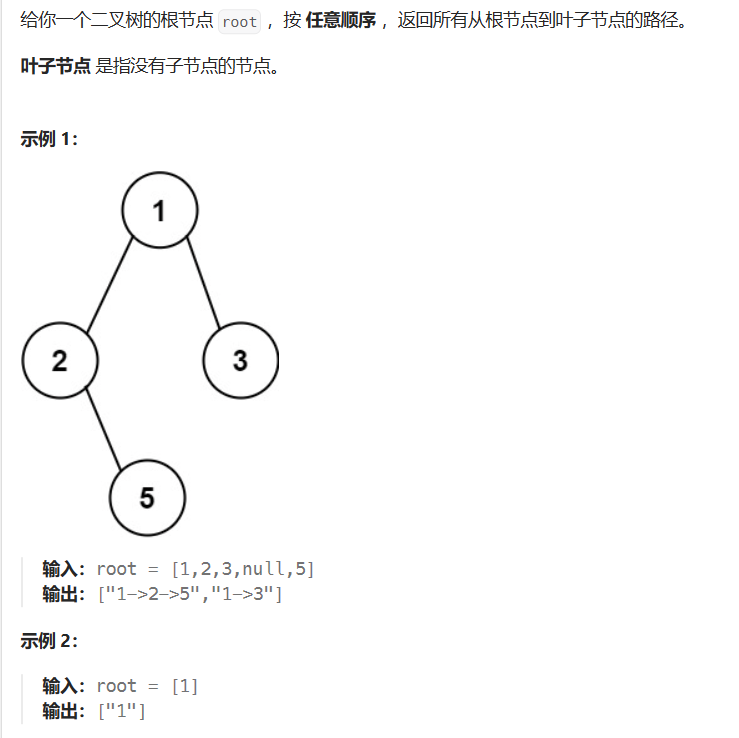
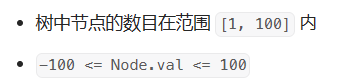
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class Solution { public: void Recursion(TreeNode* root,vector<string>& vs,string s) { if(root&&!root->left&&!root->right) { s+=(to_string(root->val)); vs.push_back(s); return; } if(!root) return; s+=(to_string(root->val)+"->"); Recursion(root->left,vs,s); Recursion(root->right,vs,s); } vector<string> binaryTreePaths(TreeNode* root) { vector<string> vs; string s; Recursion(root,vs,s); return vs; } };
|
2.6 404-左子叶之和
404
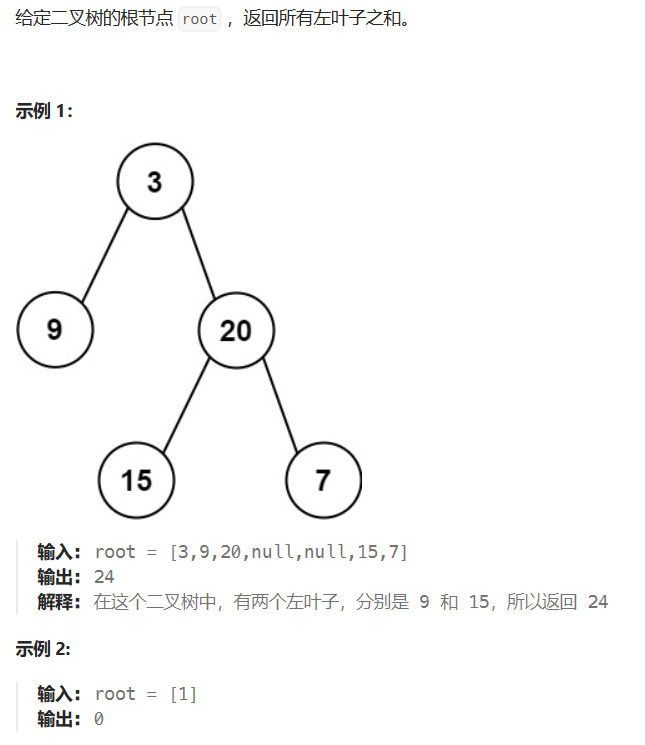
1 2 3 4 5 6 7 8 9 10 11 12
| class Solution { public: int sumOfLeftLeaves(TreeNode* root) { if (!root) return 0; int leftValue = 0; if (root->left && !root->left->left && !root->left->right) leftValue = root->left->val; return leftValue + sumOfLeftLeaves(root->left) + sumOfLeftLeaves(root->right); } };
|
2.7* 513-找树左下角的值
513
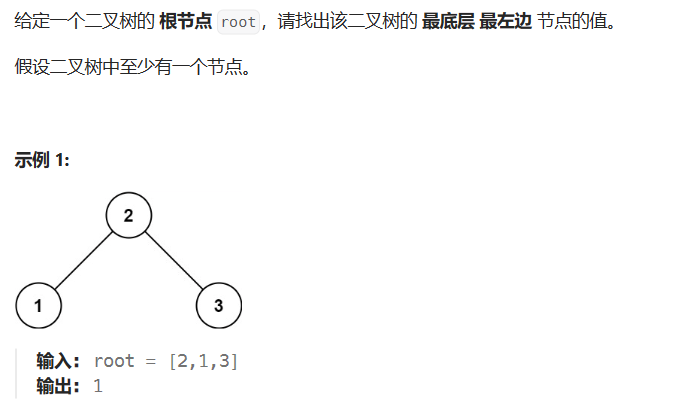
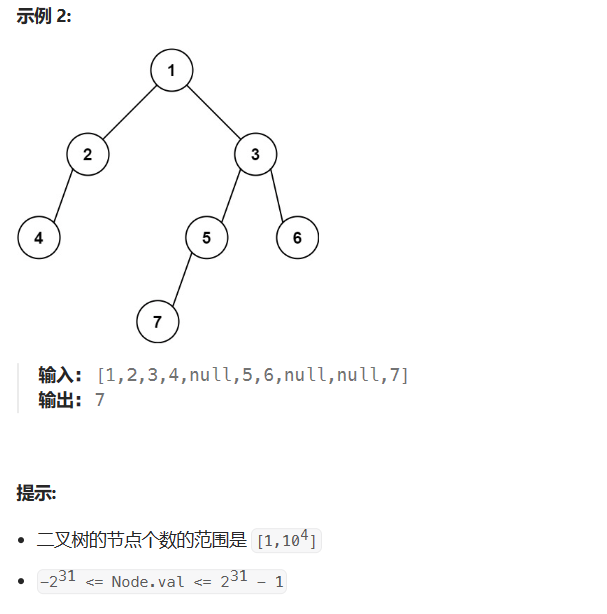
dfs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| class Solution { public: void Recursion(TreeNode* cur,int curdep,int& maxdep,int& ans) { if(!cur->left&&!cur->right) { if(curdep>maxdep) { maxdep = dep; ans = cur->val; } return; } if(cur->left) Recursion(cur->left,dep+1,maxdep,ans); if(cur->right) Recursion(cur->right,dep+1,maxdep,ans); } int findBottomLeftValue(TreeNode* root) { int ans = root->val; int maxdep = 0; Recursion(root,0,maxdep,ans); return ans; } };
|
bfs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class Solution { public: int findBottomLeftValue(TreeNode* root) { int ans = 0; queue<TreeNode*> que; que.push(root); while(!que.empty()) { TreeNode* cur = que.front(); que.pop(); if(cur->right) que.push(cur->right); if(cur->left) que.push(cur->left); ans = cur->val; } return ans; } };
|
2.8 112-路径总和
112
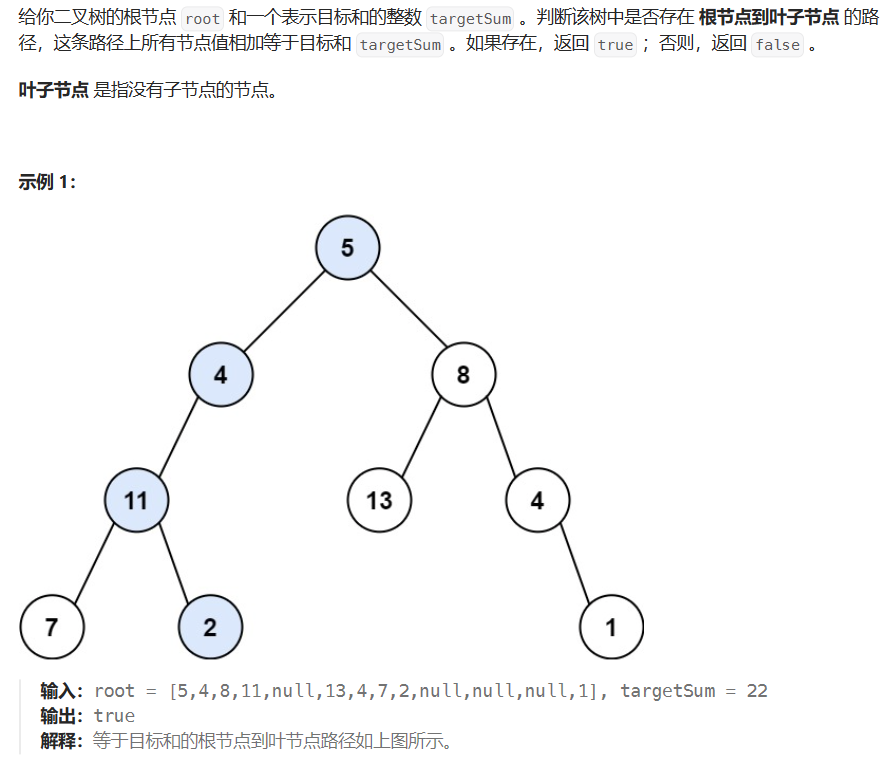
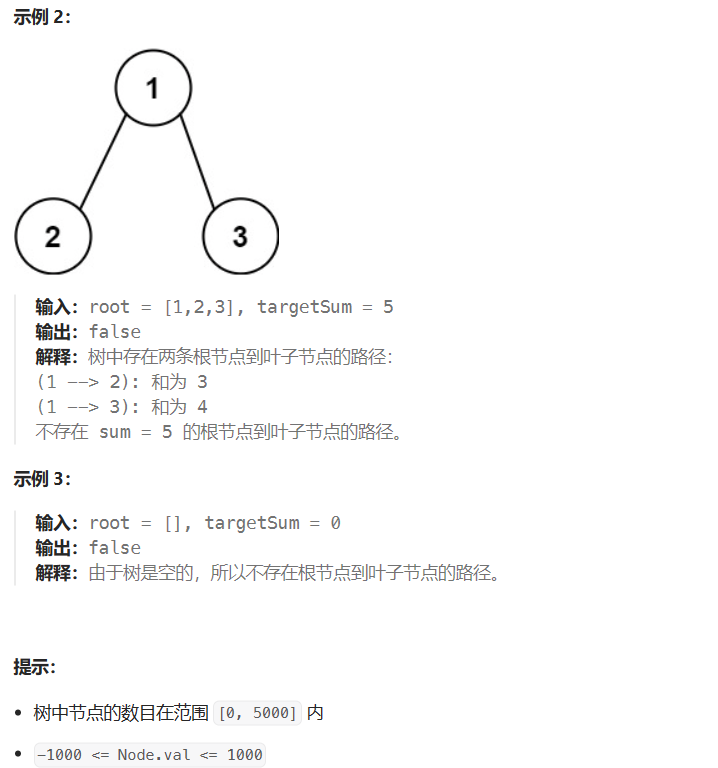
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: bool Recursion(TreeNode* cur,int sum,int target) { if(!cur) return false; if(!cur->left&&!cur->right&&sum+cur->val == target) return true; return Recursion(cur->left,sum+cur->val,target) ||Recursion(cur->right,sum+cur->val,target); } bool hasPathSum(TreeNode* root, int targetSum) { return Recursion(root,0,targetSum); } };
|
2.9* 106-从中序与后续遍历序列构造二叉树
106
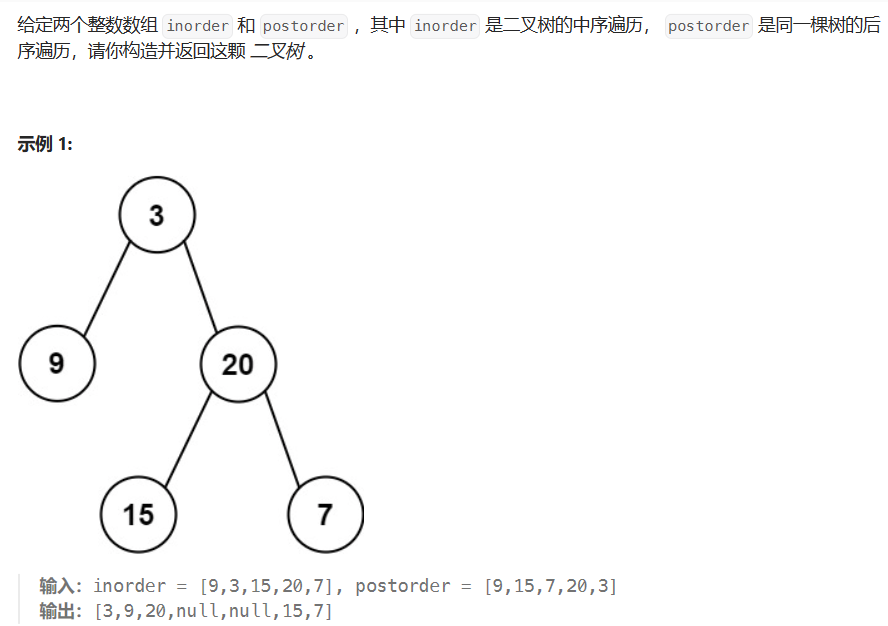
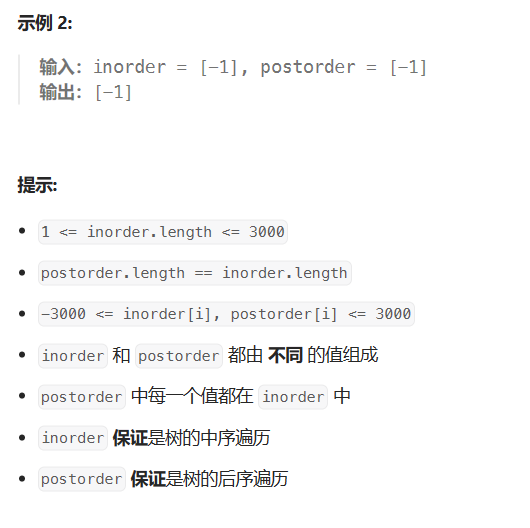
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| class Solution { public: TreeNode* Recursion(vector<int>& inorder,vector<int>& postorder,int& rootindex,int left,int right) { if(left > right) return nullptr; TreeNode* cur = new TreeNode(postorder[rootindex]); int mid = 0; for(mid = inorder.size()-1 ; mid >=0 ; --mid) if(inorder[mid] == postorder[rootindex]) break; rootindex--; cur->right = Recursion(inorder,postorder,rootindex,mid+1,right); cur->left = Recursion(inorder,postorder,rootindex,left,mid-1); return cur; } TreeNode* buildTree(vector<int>& inorder, vector<int>& postorder) { int rootindex = postorder.size() - 1; return Recursion(inorder,postorder,rootindex,0,rootindex); } };
|
2.10* 105-从前序与中序遍历序列构造二叉树
105
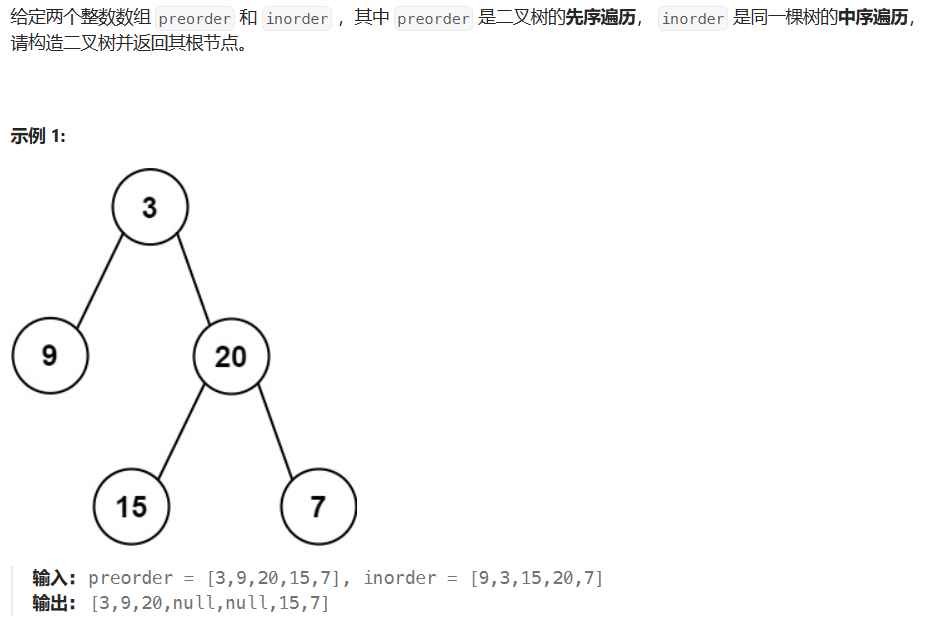
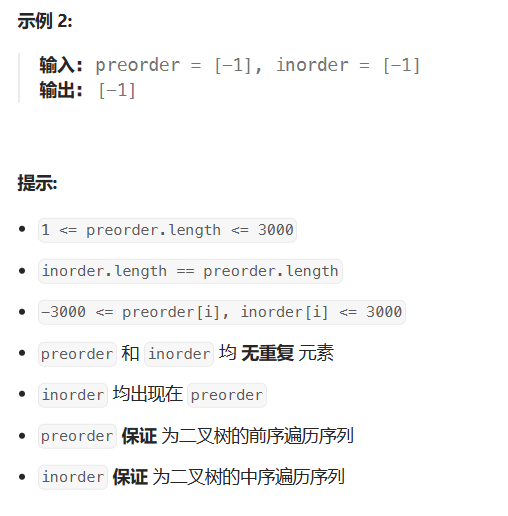
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Solution { public: TreeNode* Recursion(vector<int>& preorder, vector<int>& inorder,int& rootindex,int left , int right) { if(left > right) return nullptr; TreeNode* cur = new TreeNode(preorder[rootindex]); int mid = 0; for(mid = 0 ; mid < inorder.size() ; ++mid) if(inorder[mid] == preorder[rootindex]) break; rootindex++; cur->left = Recursion(preorder,inorder,rootindex,left,mid-1); cur->right = Recursion(preorder,inorder,rootindex,mid+1,right); return cur; } TreeNode* buildTree(vector<int>& preorder, vector<int>& inorder) { int rootindex = 0; return Recursion(preorder,inorder,rootindex,0,preorder.size()-1); } };
|
2.11* 654-最大二叉树
654
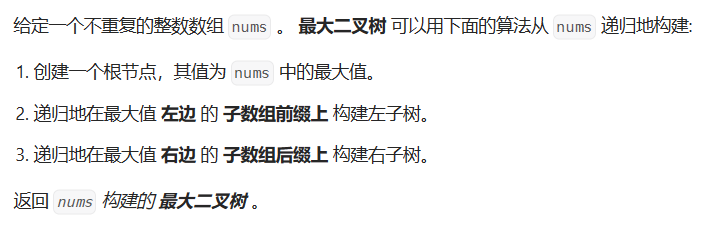
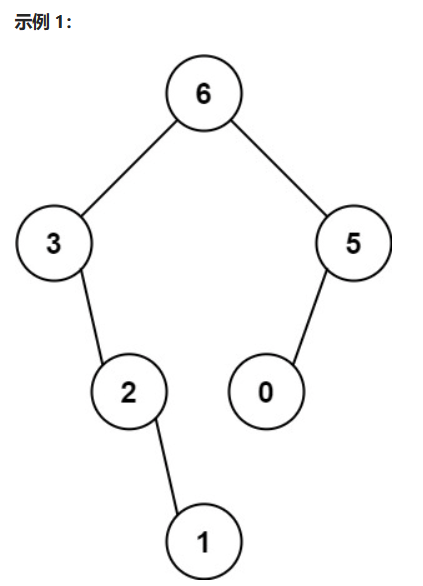
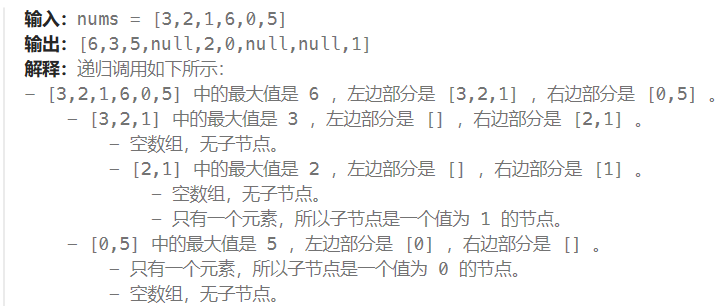
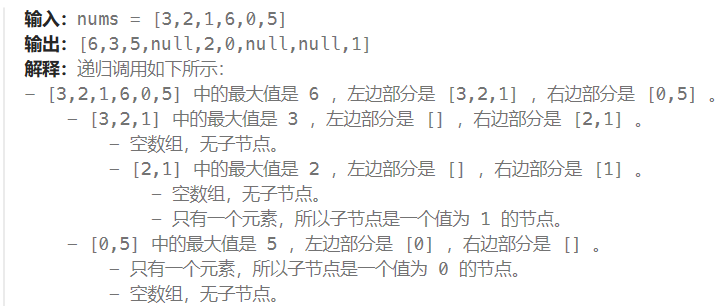
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| class Solution { public: TreeNode* Recursion(vector<int>& nums,int left,int right) { if(left > right) return nullptr; int mid = left; int max = 0; for(int i = mid ; i <= right ; ++i) { if(nums[i] > max) { mid = i; max = nums[i]; } } TreeNode* cur = new TreeNode(max); cur->left = Recursion(nums,left,mid-1); cur->right = Recursion(nums,mid+1,right); return cur; } TreeNode* constructMaximumBinaryTree(vector<int>& nums) { return Recursion(nums,0,nums.size()-1); } };
|
2.12 617-合并二叉树
617
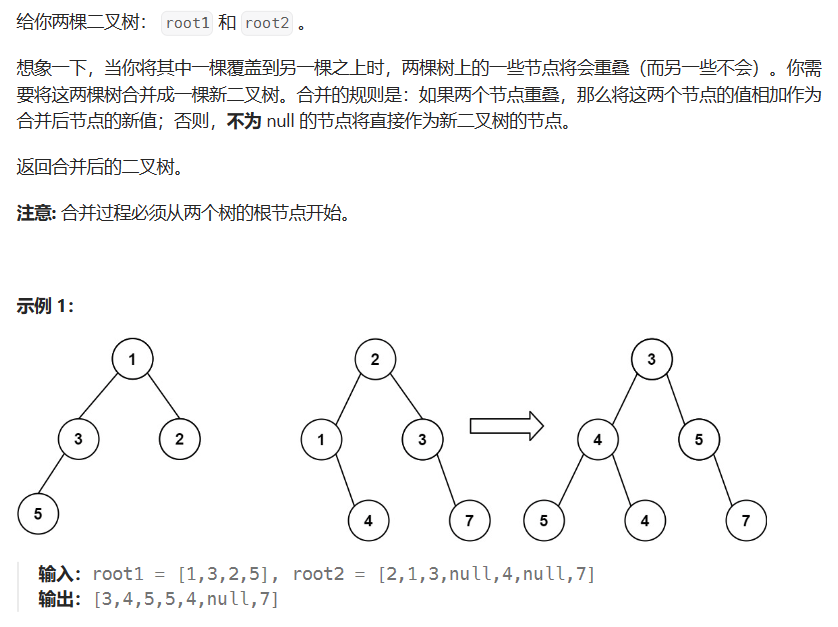
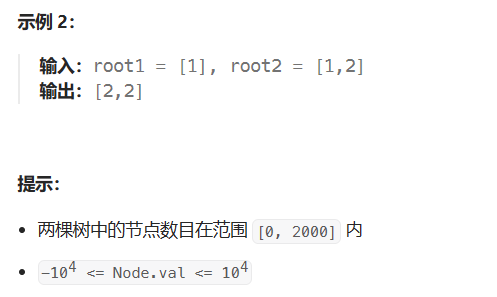
1 2 3 4 5 6 7 8 9 10 11 12 13
| class Solution { public: TreeNode* mergeTrees(TreeNode* root1, TreeNode* root2) { if(!root1) return root2; if(!root2) return root1; TreeNode* root = new TreeNode(root1->val+root2->val); root->left = mergeTrees(root1->left,root2->left); root->right = mergeTrees(root1->right,root2->right); return root; } };
|
2.13* 236-二叉树的最近公共祖先
236
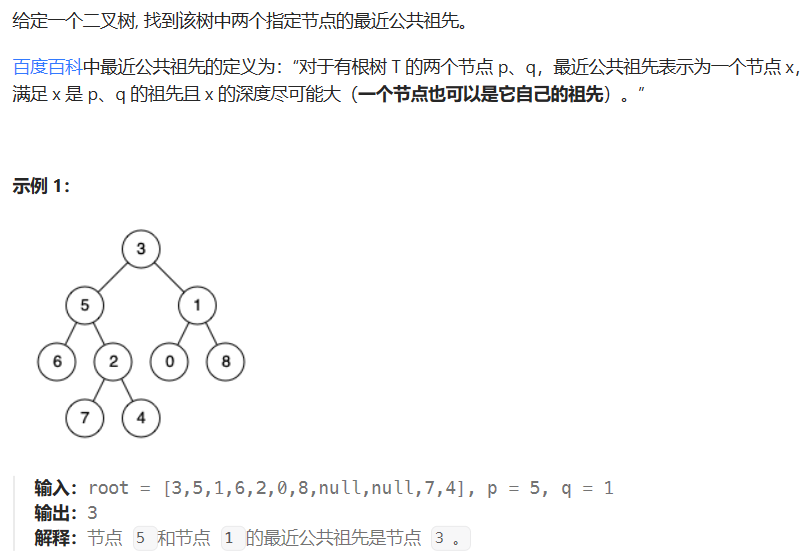
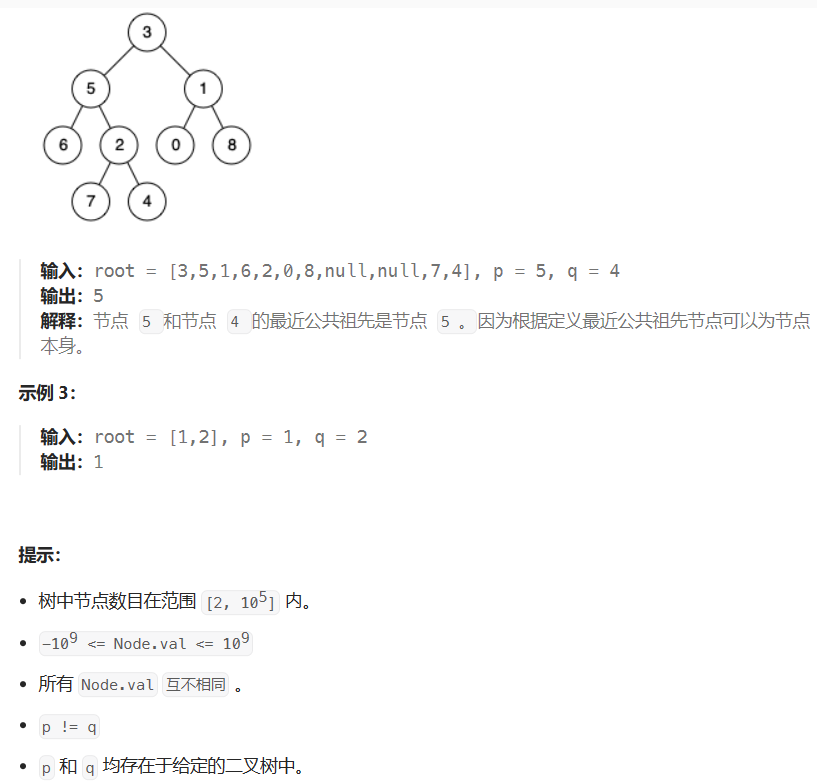
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
|
class Solution { public: TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) { if(!root) return nullptr; if(root == p || root == q) return root; TreeNode* left = lowestCommonAncestor(root->left,p,q); TreeNode* right = lowestCommonAncestor(root->right,p,q);
if(left&&right) return root; else if(!left&&right) return right; else if(left&&!right) return left; else return nullptr; } };
|
3. 二叉搜索树
3.1 700-二叉搜索树中的搜索
700
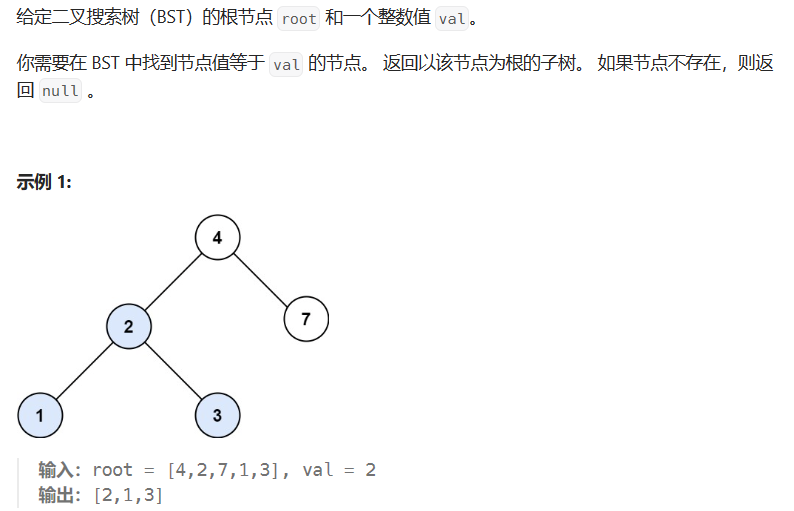
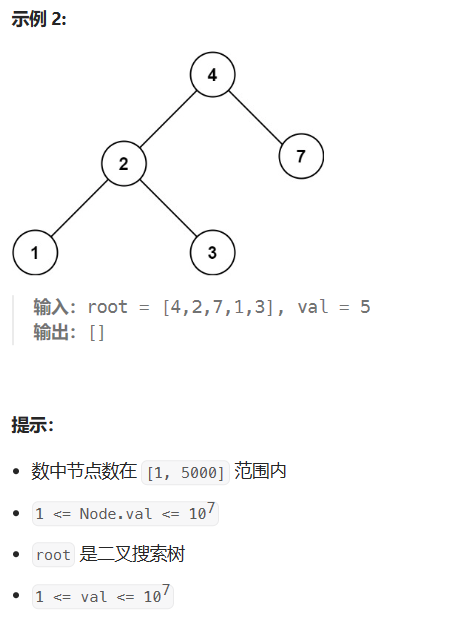
1 2 3 4 5 6 7 8 9 10 11 12 13
| class Solution { public: TreeNode* searchBST(TreeNode* root, int val) { if(!root) return nullptr; if(val < root->val) return searchBST(root->left,val); else if(val > root->val) return searchBST(root->right,val); else return root; } };
|
3.2* 98-验证二叉搜索树
98
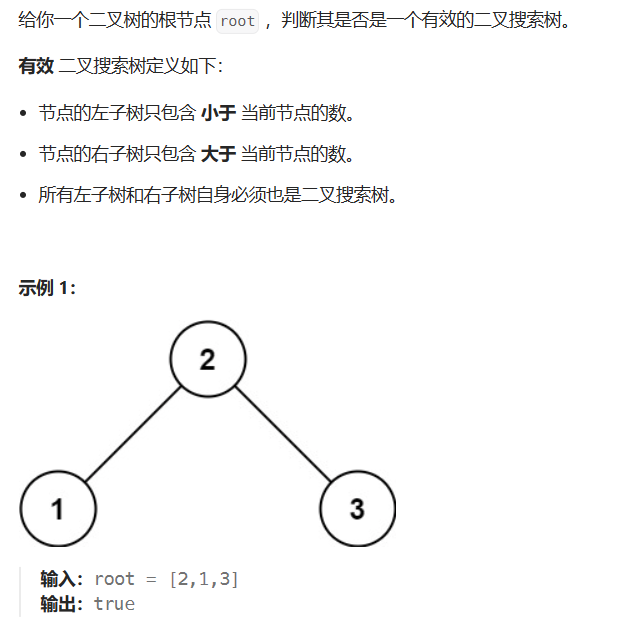
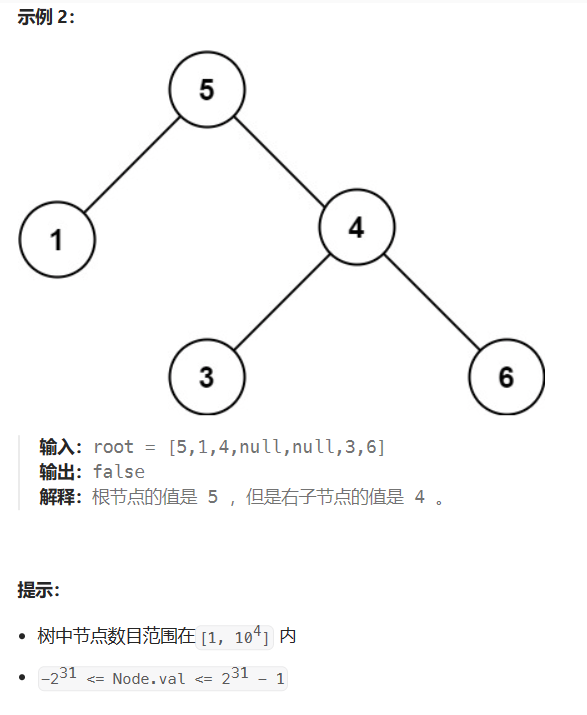
1 2 3 4 5 6 7 8 9 10 11 12 13
| class Solution { public: bool isValidBST(TreeNode* root,TreeNode* minNode = nullptr,TreeNode* maxNode = nullptr) { if(!root) return true; if((minNode && root->val <= minNode->val) || (maxNode && root->val >= maxNode->val)) return false; return isValidBST(root->left,minNode,root) && isValidBST(root->right,root,maxNode); } };
|
更直观的方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| class Solution { public: bool isValidBST(TreeNode* root) { if(!root) return true; if(root->left) { TreeNode* tmp = root->left; if(tmp->val>=root->val) return false; while(tmp->right) { tmp = tmp->right; if(tmp->val>=root->val) return false; } } if(root->right) { TreeNode* tmp = root->right; if(tmp->val<=root->val) return false; while(tmp->left) { tmp = tmp->left; if(tmp->val<=root->val) return false; } } return isValidBST(root->left) && isValidBST(root->right); } };
|
3.3 530-二叉搜索树的最小绝对差
530
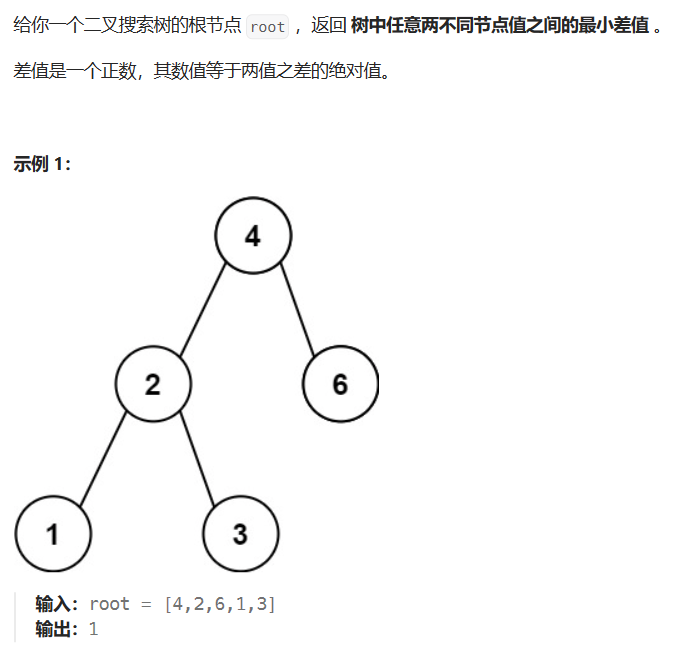
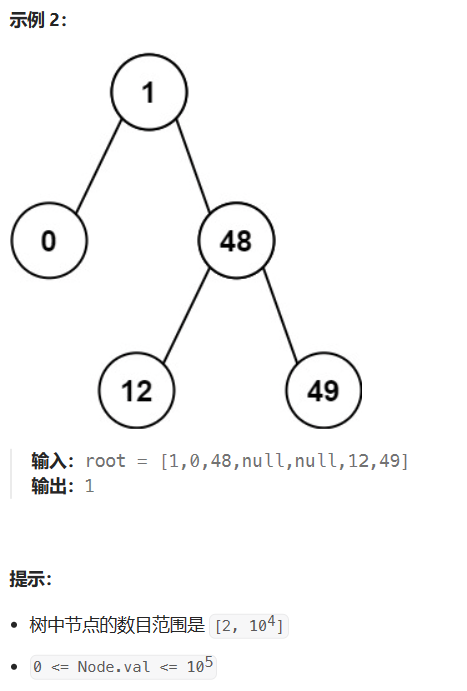
递归
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| class Solution { public: void Recursion(TreeNode* root,TreeNode*& prev,int& mindif) { if(!root) return; Recursion(root->left,prev,mindif); if(prev) mindif = min(mindif,abs(root->val-prev->val)); prev = root; Recursion(root->right,prev,mindif); } int getMinimumDifference(TreeNode* root) { int mindif = INT_MAX; TreeNode* prev = nullptr; Recursion(root,prev,mindif); return mindif; } };
|
完全遍历
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| class Solution { public: void Recursion(TreeNode* root,int& min) { if(!root) return; int tmp; if(root->left) { TreeNode* left = root->left; while(left) { tmp =abs(root->val-left->val); if(tmp<min) min = tmp; left = left->right; } } if(root->right) { TreeNode* right = root->right; while(right) { tmp = abs(root->val-right->val); if(tmp<min) min = tmp; right = right->left; } } Recursion(root->left,min); Recursion(root->right,min); } int getMinimumDifference(TreeNode* root) { int min = 100000; Recursion(root,min); return min; } };
|
3.4 501-二叉搜索树中的众数
501
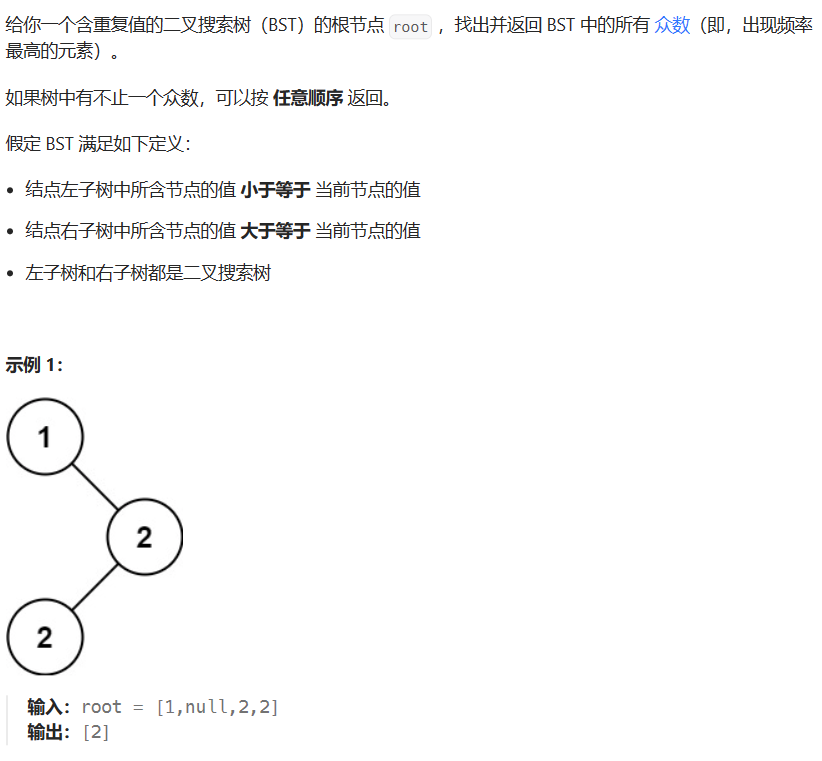
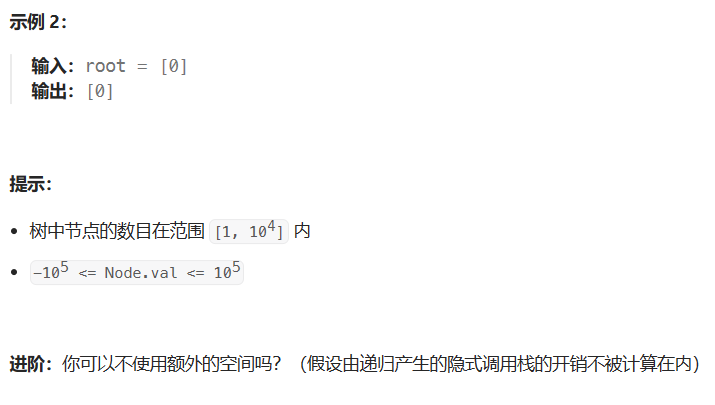
hash通解,即非二叉树也可解
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class Solution { public: void Init_um_Recursion(TreeNode* root,unordered_map<int,int>& um) { if(!root) return; um[root->val]++; Init_um_Recursion(root->left,um); Init_um_Recursion(root->right,um); } vector<int> findMode(TreeNode* root) { unordered_map<int,int> um; Init_um_Recursion(root,um);
vector<int> ans; int max = INT_MIN; for(auto& e : um) if(e.second > max) max = e.second;
for(auto& e : um) if(e.second == max) ans.push_back(e.first); return ans; } };
|
针对
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60
|
class Solution { public: int samenum = 0; int count = 0; int maxcount = 0; void Get_ret(TreeNode* root,vector<int>& ret) { if(!root) return; Get_ret(root->left,ret); ret.push_back(root->val);
if(samenum == root->val) count++; else { samenum = root->val; count=1; }
if(maxcount < count) maxcount = count; Get_ret(root->right,ret); } vector<int> findMode(TreeNode* root) { vector<int> ans; vector<int> ret; Get_ret(root,ret);
int left = 0; int right = maxcount-1; while(right < ret.size()) { if(ret[left] == ret[right]) { ans.push_back(ret[left]); left = right+1; right = left+maxcount-1; } else { left++; right++; } } return ans; } };
|
3.5* 235-二叉搜索树的最近公共祖先
235
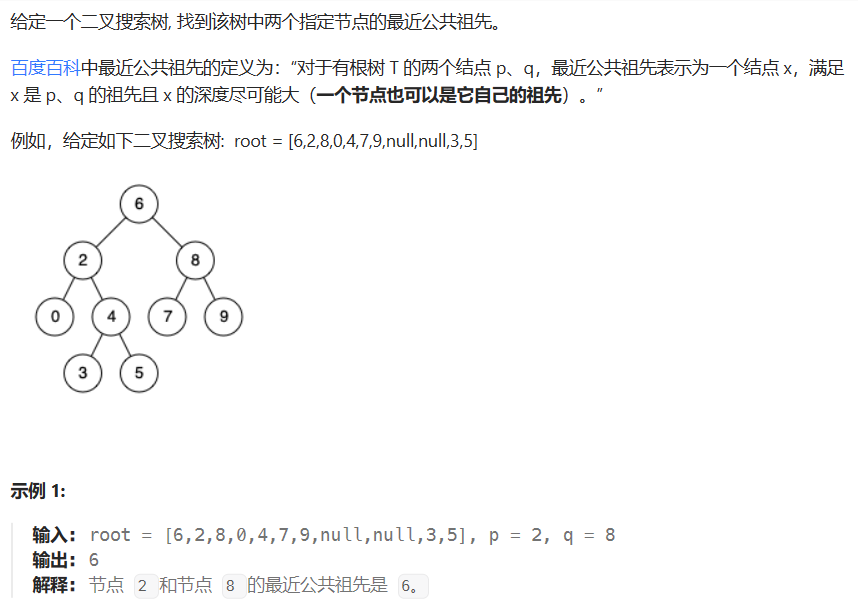
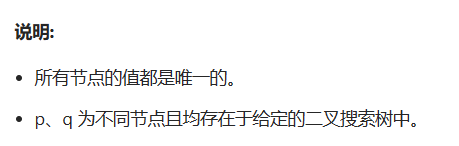
1 2 3 4 5 6 7 8 9 10 11 12 13
| class Solution { public: TreeNode* lowestCommonAncestor(TreeNode* root, TreeNode* p, TreeNode* q) { if(!root) return nullptr; if(root->val > p->val && root->val > q->val) return lowestCommonAncestor(root->left,p,q); else if(root->val<p->val&&root->val<q->val) return lowestCommonAncestor(root->right,p,q); return root; } };
|
3.6* 701-二叉搜索树中的插入操作
701
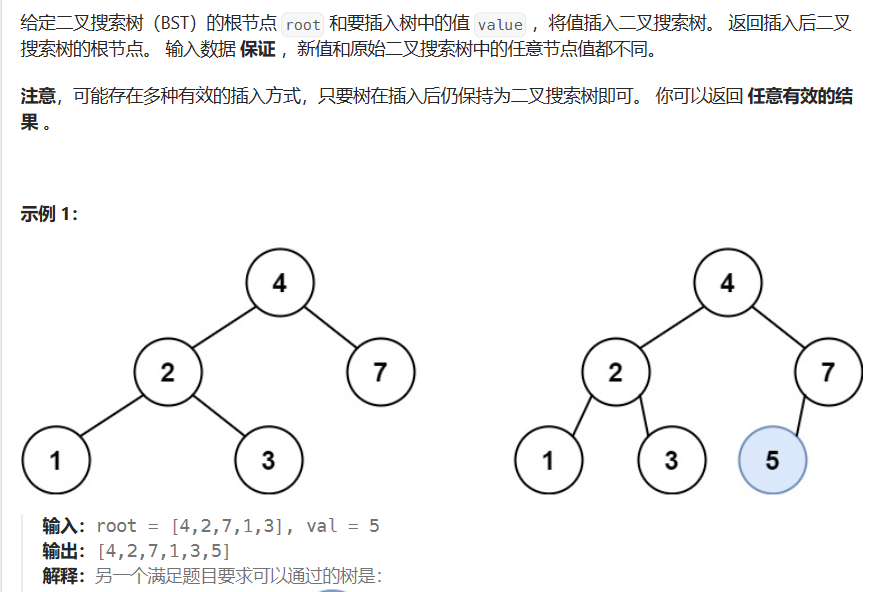
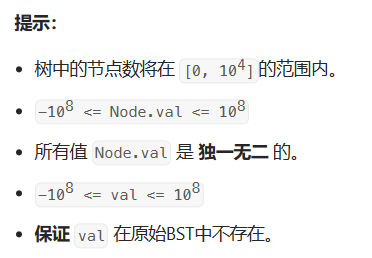
1 2 3 4 5 6 7 8 9 10 11 12
| class Solution { public: TreeNode* insertIntoBST(TreeNode* root, int val) { if(!root) return new TreeNode(val); if(val > root->val) root->right = insertIntoBST(root->right,val); else if(val < root->val) root->left = insertIntoBST(root->left,val); return root; } };
|
3.7* 450-删除二叉搜索树中的节点
450
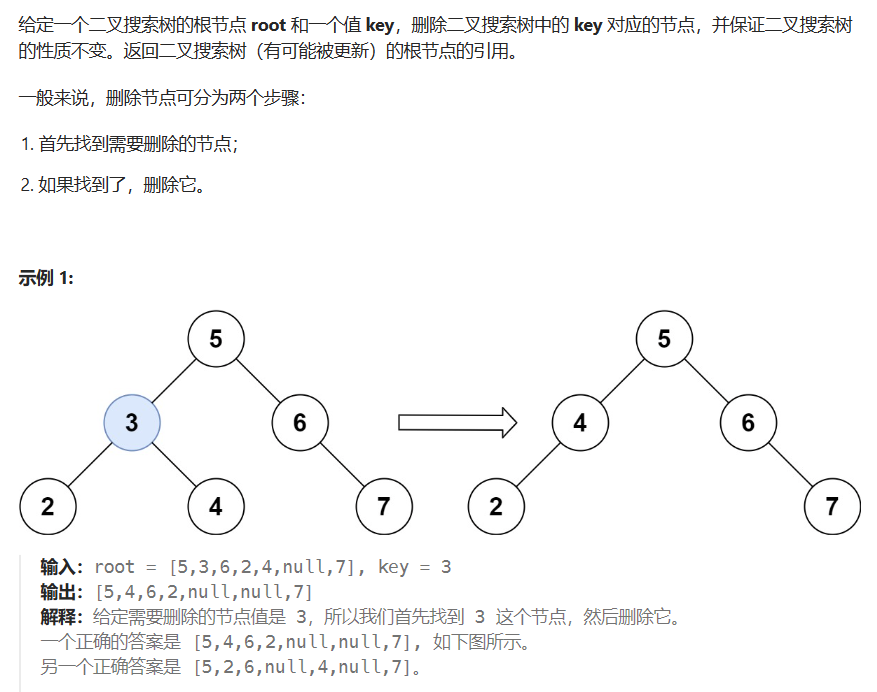
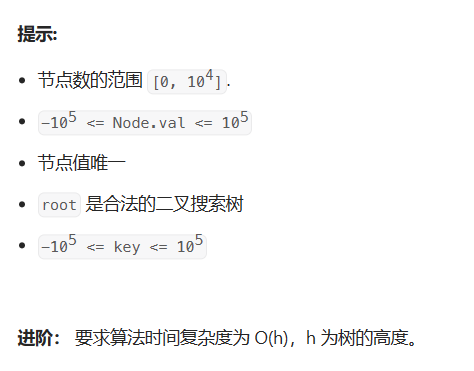
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| class Solution { public: TreeNode* findMin(TreeNode* node) { while (node->left) node = node->left; return node; }
TreeNode* deleteNode(TreeNode* root, int key) { if (!root) return root;
if (key < root->val) root->left = deleteNode(root->left, key); else if (key > root->val) root->right = deleteNode(root->right, key); else { if (!root->left) { TreeNode* temp = root->right; delete root; return temp; } else if (!root->right) { TreeNode* temp = root->left; delete root; return temp; }
TreeNode* temp = findMin(root->right); root->val = temp->val; root->right = deleteNode(root->right, temp->val); } return root; } };
|
3.8* 669-修剪二叉搜索树
669
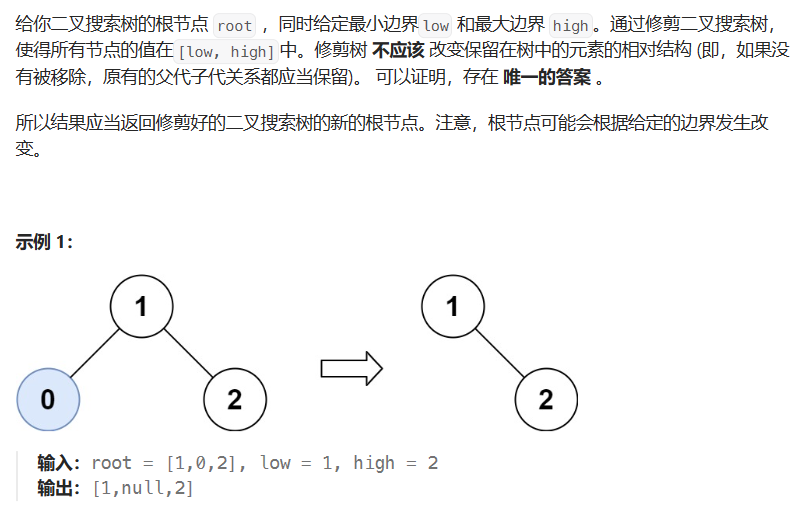
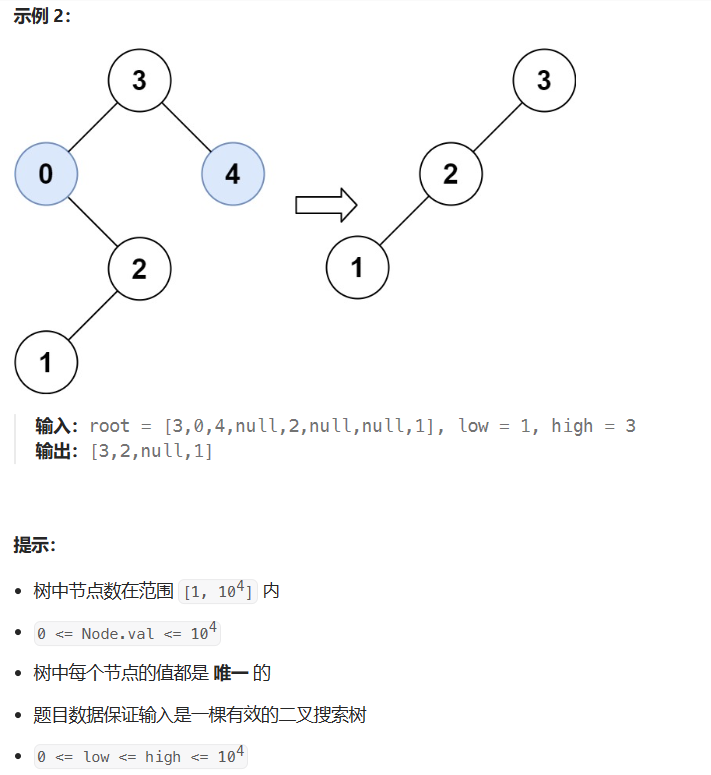
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| class Solution { public: TreeNode* trimBST(TreeNode* root, int low, int high) { if(!root) return nullptr; if(root->val<low) return trimBST(root->right,low,high); if(root->val>high) return trimBST(root->left,low,high); root->left = trimBST(root->left,low,high); root->right = trimBST(root->right,low,high); return root; } };
|
3.9 108-将有序数组转换为二叉搜索树
108
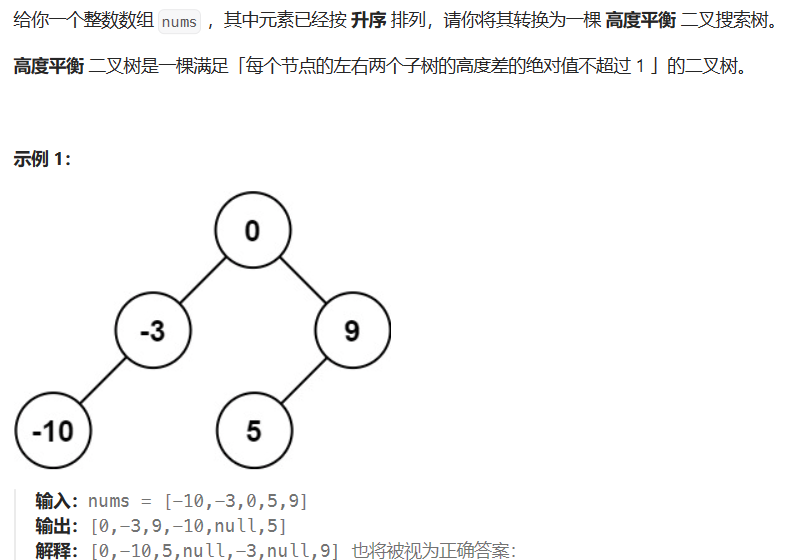
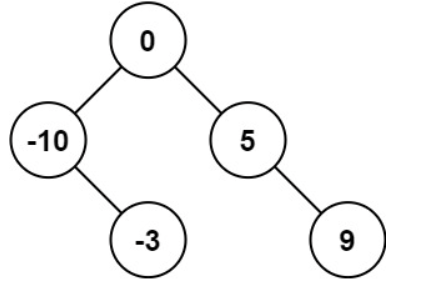
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class Solution { public: TreeNode* Recursion(vector<int>& nums,int left,int right) { if(left>right) return nullptr; int mid = left+((right-left)>>1); TreeNode* newnode = new TreeNode(nums[mid]); newnode->left = Recursion(nums,left,mid-1); newnode->right = Recursion(nums,mid+1,right); return newnode; }
TreeNode* sortedArrayToBST(vector<int>& nums) { return Recursion(nums,0,nums.size()-1); } };
|
3.10 538-把二叉搜索树转换为累加树
538
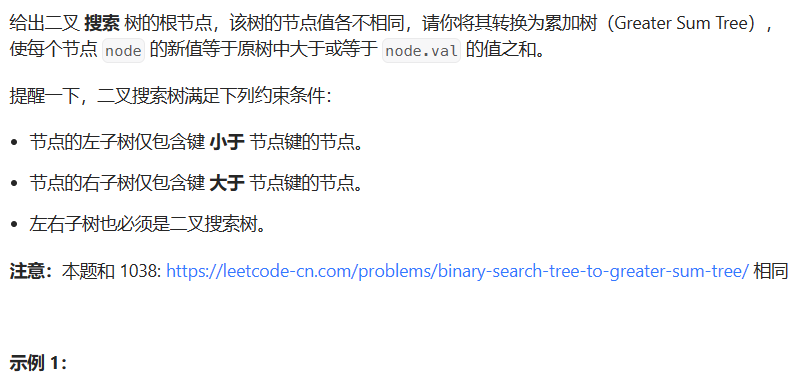
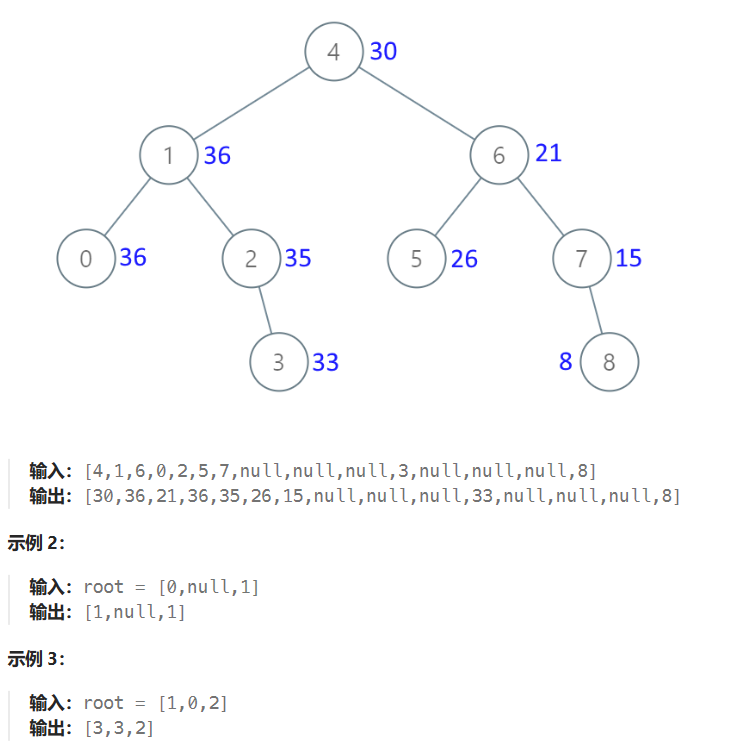
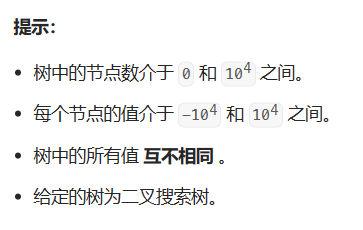
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| class Solution { public: void Recursion(TreeNode* root,int& val) { if(!root) return; Recursion(root->right,val); val+=root->val; root->val = val; Recursion(root->left,val); } TreeNode* convertBST(TreeNode* root) { int val = 0; Recursion(root,val); return root; } };
|