一、继承方式和访问方式
注意:友元不能继承
,静态成员只会在父类创造一次
父类如果用private修饰的成员,子类无法访问
protected修饰的子类可以访问,但除此之外不可访问
继承方式为private,struct为public ,一般继承方式都为public
基类的成员在子类的访问方式 = ==Min(成员在基类的访问限定符,继承方式)
二、赋值兼容规则
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| int main() { person p; student s; p = s; s = p; person* ptr = &s; person& ref = s; student* sptr = (student*)&s; sptr = (student*)&p; sptr = dynamic_cast<student*>(ptr);
return 0; }
|
三、默认函数的继承
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86
| class person { public: person(const char* name = "peter") :_name(name) { cout << "person()" << endl; }
person(const person& p) :_name(p._name) { cout << "person(const person& p)" << endl; }
person& operator=(const person& p) { cout << "person& operator=(const person& p)" << endl; if (this != &p) { _name = p._name; } return *this; }
~person() { cout << "~person()" << endl; }
protected: string _name; };
class student : public person { public: student(const char* name, int num) : person(name) , _num(num) { cout << "student()" << endl; }
student(const student& s) : person(s) ,_num(s._num) { cout << "student(const student& s)" << endl; }
student& operator=(const student& s) { cout << "student& operator=(const student& s)" << endl; if (this != &s) { person::operator=(s); _num = s._num; } return *this; }
~student() { cout << "~student()" << endl; }
private: int _num; };
int main() { student s1("jack", 18); student s2(s1); student s3("rose", 19); s1 = s3; return 0; }
|
四、不能被继承的类
1.将父类全部私有,使得子类无法调用父类的析构
2.利用final关键字
1 2 3
| class A final{public:A(){}};
class B :public A{};
|
五、菱形继承
1.菱形继承的问题
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class person{}; class student : public person{}; class teacher : public student{};
class student{}; class teacher{}; class assistant : public student,public teacher{};
class person {};
class student : public person {}; class teacher : public student {};
class assistant : public student, public teacher {};
|
菱形继承时有两个问题
数据冗余:即派生类有多份相同类型的数据
二义性: 即因为有多相同类型的数据而导致对数据处理时,不知道要处理谁
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| class person { public: string _name; };
class student :virtual public person { protected: int _num; };
class teacher :virtual public person { protected: int _id; };
class assistant : public student, public teacher { protected: string _course; };
void main() { assistant a; a._name = "peter";
a.student::_name = "xxx"; a.teacher::_name = "yyy"; }
|
2.菱形继承virtual解决的原理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| class A { public: int _a; };
class B :virtual public A { public: int _b; };
class C :virtual public A { public: int _c; };
class D : public B, public C { public: int _d; };
int main() { D d; cout << sizeof(d) << endl;
d.B::_a = 1; d.C::_a = 2; d._b = 3; d._c = 4; d._d = 5; d._a = 6; return 0; }
|
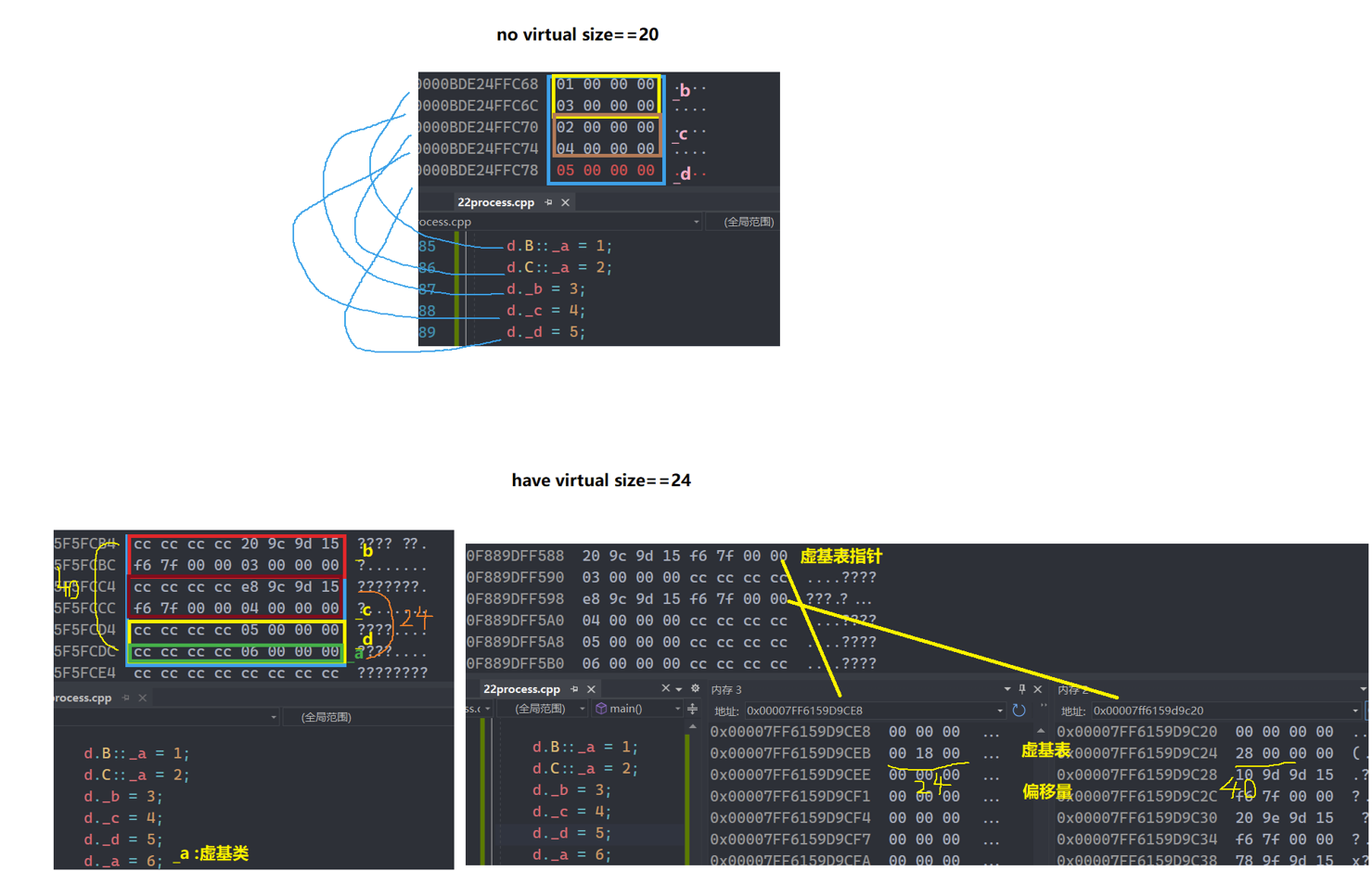
六、继承和组合
1 2 3 4 5 6 7 8 9 10 11 12 13
| class A{}; class B : public A{};
class C{}; class D {C c;};
|
一、继承方式和访问方式
注意:友元不能继承
,静态成员只会在父类创造一次
父类如果用private修饰的成员,子类无法访问
protected修饰的子类可以访问,但除此之外不可访问
继承方式为private,struct为public ,一般继承方式都为public
基类的成员在子类的访问方式 = ==Min(成员在基类的访问限定符,继承方式)
二、赋值兼容规则
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| int main() { person p; student s; p = s; s = p; person* ptr = &s; person& ref = s; student* sptr = (student*)&s; sptr = (student*)&p; sptr = dynamic_cast<student*>(ptr);
return 0; }
|
三、默认函数的继承
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86
| class person { public: person(const char* name = "peter") :_name(name) { cout << "person()" << endl; }
person(const person& p) :_name(p._name) { cout << "person(const person& p)" << endl; }
person& operator=(const person& p) { cout << "person& operator=(const person& p)" << endl; if (this != &p) { _name = p._name; } return *this; }
~person() { cout << "~person()" << endl; }
protected: string _name; };
class student : public person { public: student(const char* name, int num) : person(name) , _num(num) { cout << "student()" << endl; }
student(const student& s) : person(s) ,_num(s._num) { cout << "student(const student& s)" << endl; }
student& operator=(const student& s) { cout << "student& operator=(const student& s)" << endl; if (this != &s) { person::operator=(s); _num = s._num; } return *this; }
~student() { cout << "~student()" << endl; }
private: int _num; };
int main() { student s1("jack", 18); student s2(s1); student s3("rose", 19); s1 = s3; return 0; }
|
四、不能被继承的类
1.将父类全部私有,使得子类无法调用父类的析构
2.利用final关键字
1 2 3
| class A final{public:A(){}};
class B :public A{};
|
五、菱形继承
1.菱形继承的问题
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class person{}; class student : public person{}; class teacher : public student{};
class student{}; class teacher{}; class assistant : public student,public teacher{};
class person {};
class student : public person {}; class teacher : public student {};
class assistant : public student, public teacher {};
|
菱形继承时有两个问题
数据冗余:即派生类有多份相同类型的数据
二义性: 即因为有多相同类型的数据而导致对数据处理时,不知道要处理谁
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| class person { public: string _name; };
class student :virtual public person { protected: int _num; };
class teacher :virtual public person { protected: int _id; };
class assistant : public student, public teacher { protected: string _course; };
void main() { assistant a; a._name = "peter";
a.student::_name = "xxx"; a.teacher::_name = "yyy"; }
|
2.菱形继承virtual解决的原理
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
| class A { public: int _a; };
class B :virtual public A { public: int _b; };
class C :virtual public A { public: int _c; };
class D : public B, public C { public: int _d; };
int main() { D d; cout << sizeof(d) << endl;
d.B::_a = 1; d.C::_a = 2; d._b = 3; d._c = 4; d._d = 5; d._a = 6; return 0; }
|

六、继承和组合
1 2 3 4 5 6 7 8 9 10 11 12 13
| class A{}; class B : public A{};
class C{}; class D {C c;};
|